C++ Exercises: Check whether two straight lines AB and CD are orthogonal or not
Orthogonal Lines Check
There are four different points on a plane: A(x1, y1), B(x2, y2), C(x3, y3) and D(x4, y4).
Write a C++ program to check whether two straight lines AB and CD are orthogonal or not.
Input:
0 6
5 6
3 8
3 2
Output:
yes
Visual Presentation:
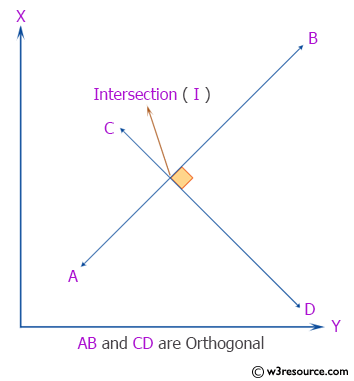
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
int main(void) {
double x[4], y[4]; // Arrays to store x and y coordinates of points
// Continuously take input for x and y coordinates until EOF
while (cin >> x[0] >> y[0]) {
// Input coordinates of points
for (int i = 1; i < 4; i++) {
cin >> x[i] >> y[i];
}
// Check if vectors AB and CD are orthogonal using dot product
if ((x[1] - x[0]) * (x[3] - x[2]) + (y[3] - y[2]) * (y[1] - y[0]) == 0) {
cout << "Yes, AB and CD are orthogonal." << endl; // Output if AB and CD are orthogonal
} else {
cout << "Yes, AB and CD are not orthogonal." << endl; // Output if AB and CD are not orthogonal
}
}
return 0;
}
Sample Output:
Input number: 0 6 5 6 3 8 3 2 Yes, AB and CD are orthogonal.
Flowchart:
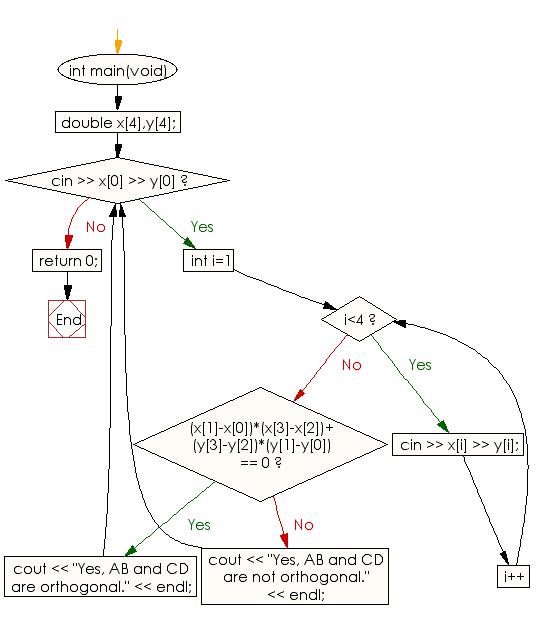
For more Practice: Solve these Related Problems:
- Write a C++ program to check whether two lines defined by points A, B and C, D are perpendicular using vector dot product.
- Write a C++ program that reads coordinates for four points and determines if line AB is orthogonal to line CD with appropriate precision.
- Write a C++ program to determine if two lines are perpendicular by calculating the slopes and verifying that their product is -1.
- Write a C++ program that uses user-defined functions to check the orthogonality of two lines based on coordinate geometry.
C++ Code Editor:
Previous: Write a C++ program that accept an integer (n) from the user and outputs the number of combinations that express n as a sum of two prime numbers.
Next: Write a C++ program to sum of all positive integers in a sentence.
What is the difficulty level of this exercise?