C++ Exercises: Find the number and sum of all integer between 100 and 200 which are divisible by 9
28. Sum of Integers Between 100 and 200 Divisible by 9
Write a program in C++ to find the number and sum of all integers between 100 and 200 which are divisible by 9.
Visual Presentation:
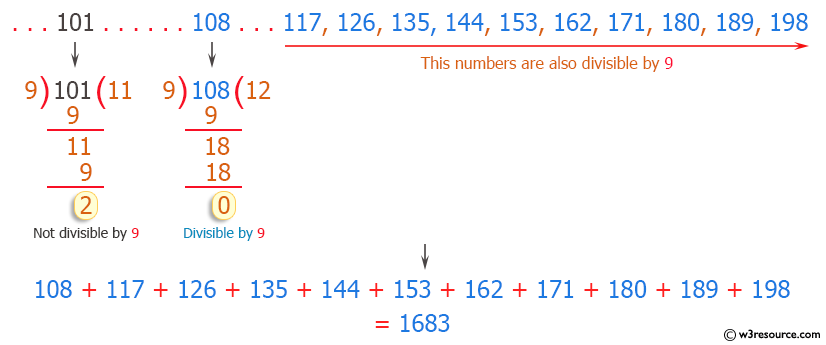
Sample Solution:-
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
int i, sum = 0; // Declaration of integer variables 'i' (loop counter), 'sum' (stores the sum of numbers)
cout << "\n\n Find the number and sum of all integers between 100 and 200, divisible by 9:\n"; // Displaying a message on the console
cout << "--------------------------------------------------------------------------------\n";
cout << " Numbers between 100 and 200, divisible by 9: " << endl; // Displaying a message indicating the range of numbers
for (i = 101; i < 200; i++) // Loop to iterate through numbers between 101 and 199 (inclusive)
{
if (i % 9 == 0) // Checking if the number is divisible by 9
{
cout << " " << i; // Displaying the number if it is divisible by 9
sum += i; // Adding the number to the sum
}
}
cout << "\n The sum : " << sum << endl; // Displaying the sum of the numbers divisible by 9
}
Sample Output:
Find the number and sum of all integer between 100 and 200, divisible by 9: -------------------------------------------------------------------------------- Numbers between 100 and 200, divisible by 9: 108 117 126 135 144 153 162 171 180 189 198 The sum : 1683
Flowchart:
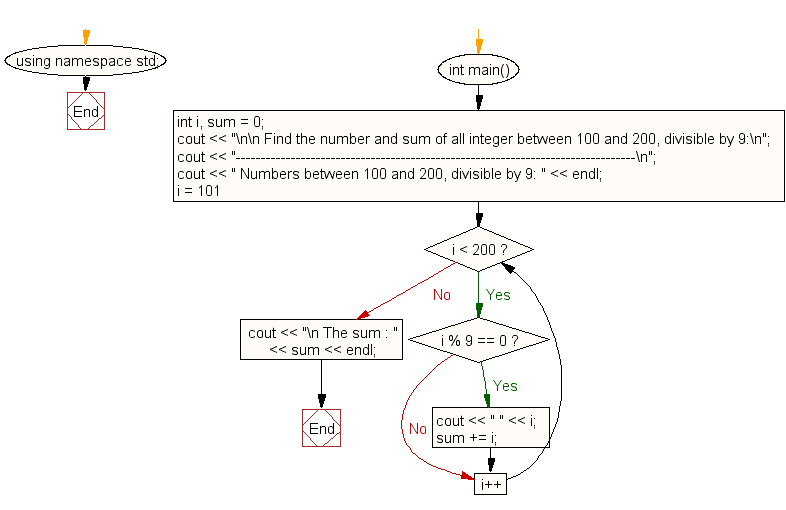
For more Practice: Solve these Related Problems:
- Write a C++ program to list and sum all integers between 100 and 200 that are divisible by 9 using a for loop.
- Write a C++ program that reads a range and outputs the numbers divisible by 9 within that range and their total sum.
- Write a C++ program to check each number from 100 to 200 for divisibility by 9 and print the numbers along with the sum.
- Write a C++ program that generates the sequence of numbers divisible by 9 in a specified range and computes their aggregate.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the first n terms of Fibonacci series.
Next: Write a program in C++ to find LCM of any two numbers using HCF.
What is the difficulty level of this exercise?