C++ Exercises: Display n terms of natural number and their sum
3. Display n Terms of Natural Numbers and Their Sum
Write a program in C++ to display n terms of natural numbers and their sum.
Visual Presentation:
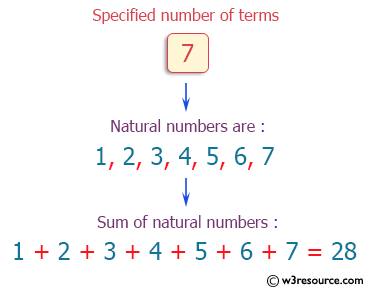
Sample Solution :-
C++ Code :
#include <iostream> // Preprocessor directive to include the input/output stream header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int n, i, sum = 0; // Declare integer variables 'n', 'i', and 'sum' and initialize 'sum' to 0
cout << "\n\n Display n terms of natural number and their sum:\n"; // Display a message indicating the purpose
cout << "---------------------------------------\n"; // Display a separator line
cout << " Input a number of terms: "; // Prompt the user to input the number of terms
cin >> n; // Read the user input for the number of terms
cout << " The natural numbers up to the " << n << "th term are: \n"; // Display a message indicating the list of natural numbers up to 'n'
// Loop to print the natural numbers up to 'n' and calculate their sum
for (i = 1; i <= n; i++)
{
cout << i << " "; // Output each natural number followed by a space
sum = sum + i; // Calculate the sum by adding the current number 'i' to 'sum'
}
cout << "\n The sum of the natural numbers is: " << sum << endl; // Display the sum of the natural numbers
}
Sample Output:
Display n terms of natural number and their sum: --------------------------------------- Input a number of terms: 7 The natural numbers upto 7th terms are: 1 2 3 4 5 6 7 The sum of the natural numbers is: 28
Flowchart:
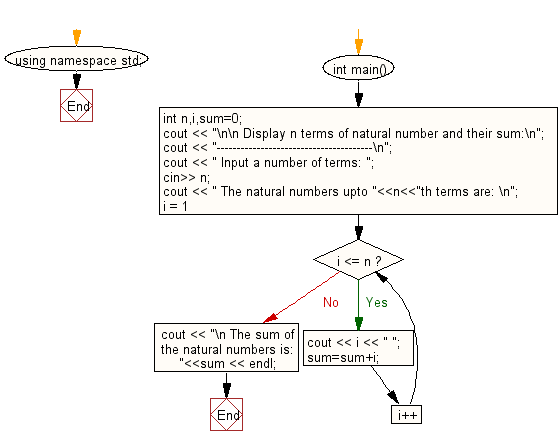
For more Practice: Solve these Related Problems:
- Write a C++ program to print the first n natural numbers and calculate their sum using a for loop with dynamic input.
- Write a C++ program to display natural numbers up to n using recursion and then compute their total.
- Write a C++ program that prompts for n and then prints the sequence of natural numbers along with a running total on each line.
- Write a C++ program to generate an array of the first n natural numbers and then compute and display the sum of its elements.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of first 10 natural numbers.
Next: Write a program in C++ to find the perfect numbers between 1 and 500.
What is the difficulty level of this exercise?