C++ Exercises: Calculate the sum of the series 1.2+2.3+3.4+4.5+5.6+.....
67. Sum of Series 1.2 + 2.3 + 3.4 + ...
Write a program in C++ to calculate the sum of the series 1.2+2.3+3.4+4.5+5.6+.......
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int trm; // Declare an integer variable 'trm' to store the last term of the series
double num, sum, i, m; // Declare double variables for calculations: num, sum, i (iteration), and m (multiplier)
// Display a message to calculate the sum of a specific series
cout << "\n\n calculate the sum of the series 1.2+2.3+3.4+4.5+5.6+......:\n";
cout << "----------------------------------------------------------------\n";
cout << " Input the last integer between 1 to 98 without fraction you want to add: ";
cin >> trm; // Input the last term from the user
sum = 0; // Initialize the sum to zero before starting the series calculation
// Loop to calculate the series and print individual elements
for (i = 1; i <= trm; i++)
{
if (i < 9)
{
m = .1; // Set the multiplier 'm' based on the value of 'i'
}
else
{
m = .01; // Set the multiplier 'm' based on the value of 'i'
}
num = i + ((i + 1) * (m)); // Calculate each term of the series
sum = sum + num; // Add the current term to the running sum
cout << num; // Display the current term of the series
if (i < trm)
{
cout << " + "; // Display '+' between terms except for the last one
}
}
cout << "\n The sum of the series = " << sum << endl; // Display the final sum of the series
}
Sample Output:
calculate the sum of the series 1·2+2·3+3·4+4.5+5.6+......: ---------------------------------------------------------------- Input the last integer between 1 to 98 without fraction you want to ad d: 10 1.2 + 2.3 + 3.4 + 4.5 + 5.6 + 6.7 + 7.8 + 8.9 + 9.1 + 10.11 The sum of the series =59.61
Flowchart:
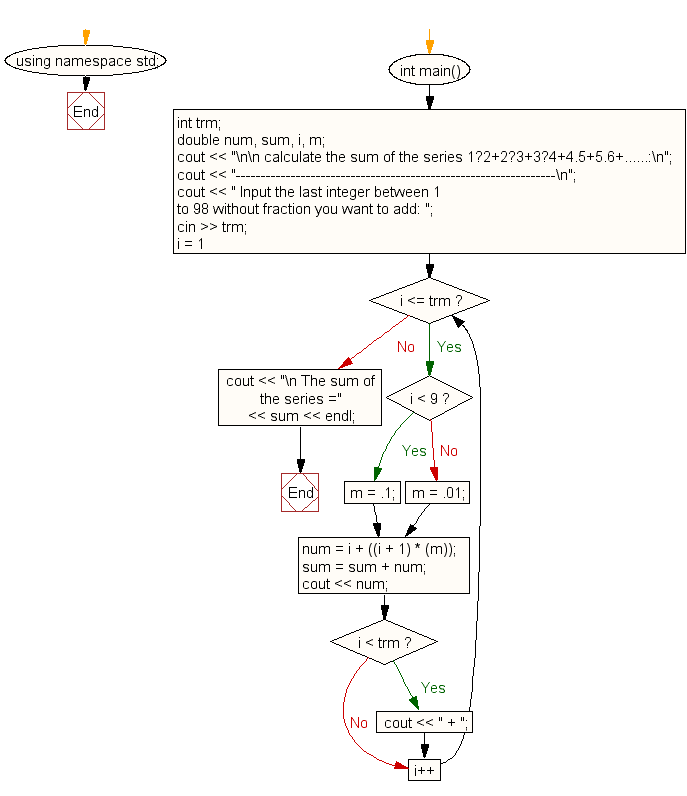
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of the series where each term is of the form n.(n+1) divided by 10, for n terms.
- Write a C++ program that reads an integer and calculates the sum of the series: 1.2, 2.3, 3.4, ... using a loop.
- Write a C++ program to iterate through numbers and sum the series defined by adding a decimal increment to each integer.
- Write a C++ program that computes a series sum where each term is formed by concatenating the number with its successor as a decimal value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write code to create a checkerboard pattern with the words "black" and "white".
Next: Write a program that will print the first N numbers for a specific base.
What is the difficulty level of this exercise?