C++ Exercises: Produce a square matrix with 0's down the main diagonal
C++ For Loop: Exercise-69 with Solution
Write a program in C++ to produce a square matrix with 0's down the main diagonal, 1's in the
entries just above and below the main diagonal, 2's above and below that, etc.
0 1 2 3 4
1 0 1 2 3
2 1 0 1 2
3 2 1 0 1
4 3 2 1 0
Visual Presentation:
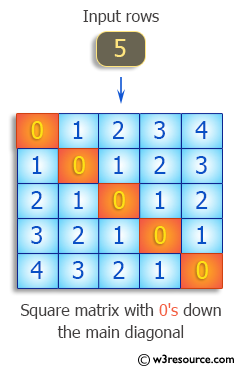
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int n, i, j, k, m = 0; // Declare integer variables 'n', 'i', 'j', 'k', 'm' and initialize 'm' as 0
cout << "\n\n Print patern........:\n"; // Display message prompting for pattern printing
cout << "-----------------------------------\n"; // Display separator line
cout << " Input number or rows: "; // Prompt user to input the number of rows for the pattern
cin >> n; // Read the number of rows input by the user
for (i = 1; i <= n; i++) { // Outer loop iterating through each row from 1 to 'n'
if (i == 1) { // For the first row,
for (j = 1; j <= i; j++) { // Loop to print 'm' (which is 0) once
cout << m << " ";
}
for (k = 1; k <= n - i; k++) { // Loop to print numbers from 1 to (n - i)
cout << k << " ";
}
}
else { // For rows other than the first row,
for (k = i - 1; k >= 1; k--) { // Loop to print numbers in decreasing order from (i - 1) to 1
cout << k << " ";
}
cout << m << " "; // Print 'm' (which is 0) once in the middle of the row
for (j = 1; j <= n - i; j++) { // Loop to print numbers from 1 to (n - i)
cout << j << " ";
}
}
cout << endl; // Move to the next line after printing each row
}
cout << endl; // Add an extra newline at the end
}
Sample Output:
Print patern........: ----------------------------------- Input number or rows: 8 0 1 2 3 4 5 6 7 1 0 1 2 3 4 5 6 2 1 0 1 2 3 4 5 3 2 1 0 1 2 3 4 4 3 2 1 0 1 2 3 5 4 3 2 1 0 1 2 6 5 4 3 2 1 0 1 7 6 5 4 3 2 1 0
Flowchart:
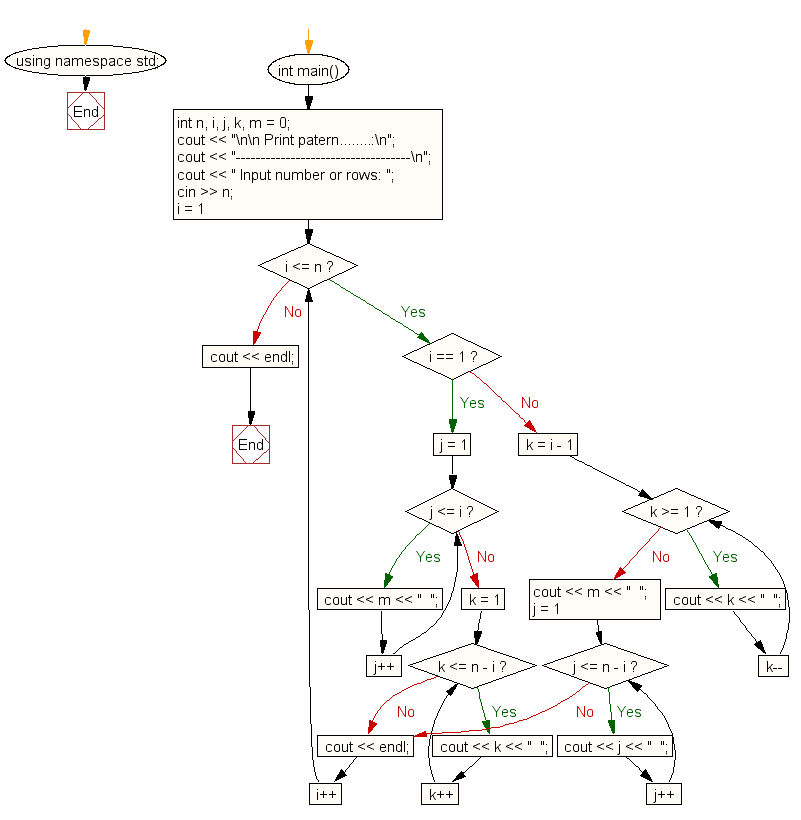
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program that will print the first N numbers for a specific base.
Next: Write a program in C++ to convert a decimal number to binary number.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-69.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics