C++ Exercises: Find the factorial of a number
7. Find the Factorial of a Number
Write a program in C++ to find the factorial of a number.
Visual Presentation:
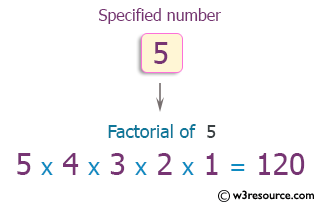
Sample Solution :-
C++ Code :
#include <iostream> // Preprocessor directive to include the input/output stream header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int num1, factorial = 1; // Declaration of integer variables 'num1' and 'factorial' initialized to 1
cout << "\n\n Find the factorial of a number:\n"; // Display a message indicating the purpose
cout << "------------------------------------\n"; // Display a separator line
cout << " Input a number to find the factorial: "; // Prompting the user to input a number
cin >> num1; // Reading the number entered by the user
for (int a = 1; a <= num1; a++) // Loop to calculate the factorial
{
factorial = factorial * a; // Calculating factorial by multiplying 'factorial' with 'a'
}
cout << " The factorial of the given number is: " << factorial << endl; // Displaying the factorial of the entered number
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the factorial of a number: ------------------------------------ Input a number to find the factorial: 5 The factorial of the given number is: 120
Flowchart:
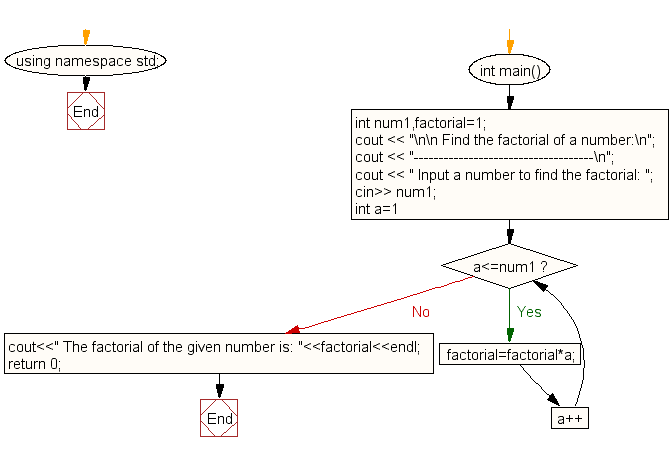
For more Practice: Solve these Related Problems:
- Write a C++ program to calculate the factorial of a number using both recursion and iteration.
- Write a C++ program that computes the factorial of an input number and outputs the multiplication sequence.
- Write a C++ program to find the factorial of a number using a while loop and display the result.
- Write a C++ program to compute the factorial of a number and check for overflow for large inputs.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find prime number within a range.
Next: Write a program in C++ to find the last prime number occur before the entered number.
What is the difficulty level of this exercise?