C++ Linked List Exercises: Delete the last node of a Singly Linked List
C++ Linked List: Exercise-12 with Solution
Write a C++ program to delete the last node of a Singly Linked List.
Test Data:
Original list:
7 5 3 1
Remove the last node of the said list:
Updated list:
7 5 3
Again remove the last node of the said list:
Updated list:
7 5
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
struct Node // Declare a structure for defining a Node
{
int num; // Data field to store a number
Node *next; // Pointer to the next node
}; // Node constructed
int sizen = 0; // Initializing variable to keep track of the size of the linked list
// Function to insert a node at the start of the linked list
void insert(Node** head, int num){
Node* new_Node = new Node(); // Creating a new node
new_Node->num = num; // Assigning data to the new node
new_Node->next = *head; // Pointing the new node to the current head
*head = new_Node; // Making the new node as the head
sizen++; // Increasing the size of the linked list
}
// Function to delete the last node of the linked list
Node* delete_last_node(struct Node* head) {
if (head == NULL) // Base case: if the list is empty
return NULL;
if (head->next == NULL) { // Base case: if there's only one node in the list
delete head; // Delete the head node
return NULL;
}
Node* second_last_node = head; // Locate the second last node
while (second_last_node->next->next != NULL)
second_last_node = second_last_node->next;
delete (second_last_node->next); // Remove the last node from the list
second_last_node->next = NULL; // Change the next of the second last node to NULL
return head; // Return the updated head
}
// Function to display all nodes in the linked list
void display_all_nodes(Node* node) {
while (node != NULL) { // Loop through all nodes until the end is reached
cout << node->num << " "; // Displaying the data in the current node
node = node->next; // Move to the next node
}
}
int main() {
Node* head = NULL; // Initializing the head of the linked list as NULL
insert(&head,1); // Inserting a node with value 1
insert(&head,3); // Inserting a node with value 3
insert(&head,5); // Inserting a node with value 5
insert(&head,7); // Inserting a node with value 7
cout << "Original list:\n"; // Displaying message for the original list
display_all_nodes(head); // Displaying all nodes in the original list
cout << "\n\nRemove the last node of the said list:"; // Displaying message for removal of last node
cout << "\nUpdated list:\n";
head = delete_last_node(head); // Removing the last node of the list
display_all_nodes(head); // Displaying all nodes after removal
cout << "\n\nAgain remove the last node of the said list:"; // Displaying message for removal of last node again
cout << "\nUpdated list:\n";
head = delete_last_node(head); // Removing the last node of the list again
display_all_nodes(head); // Displaying all nodes after removal
cout << endl; // Displaying newline
return 0; // Returning from the main function
}
Sample Output:
Original list: 7 5 3 1 Remove the last node of the said list: Updated list: 7 5 3 Again remove the last node of the said list: Updated list: 7 5
Flowchart:
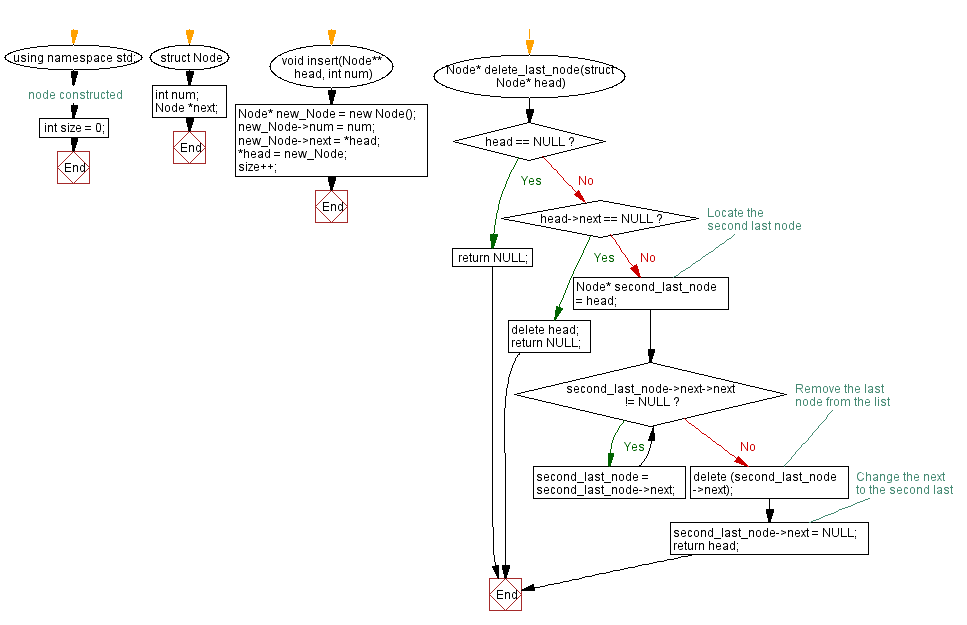
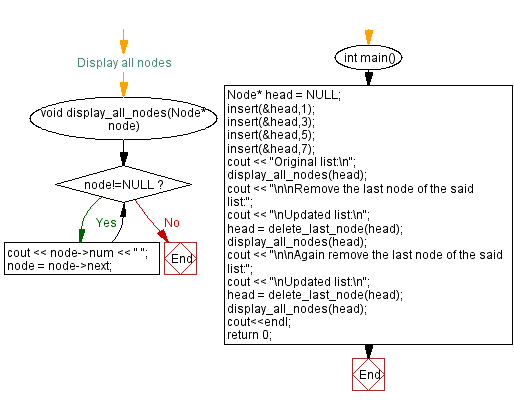
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Delete a middle node from a Singly Linked List.
Next C++ Exercise: Delete the nth node of a Singly Linked List from end.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics