C++ Linked List Exercises: Create and display a doubly linked list
15. Create and Display Doubly Linked List
Write a C++ program to create and display a doubly linked list.
Test Data:
Doubly linked list is as follows:
Traversal in Forward direction:
Orange White Green Red
Traversal in Reverse direction:
Red Green White Orange
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// A doubly linked list node
struct Node {
string data; // Data field to store string data
struct Node* next; // Pointer to the next node
struct Node* prev; // Pointer to the previous node
};
// Function to append data at the front of the doubly linked list
void append_data(Node** head, string new_data)
{
// Create a new node and allocate memory.
struct Node* newNode = new Node;
// Assign data to the new node
newNode->data = new_data;
// A new node has been added with the name head and the previous node
// set to null, since it is being added at the front.
newNode->next = (*head);
newNode->prev = NULL;
// Previous head is the new node
if ((*head) != NULL)
(*head)->prev = newNode;
// Head points to new node
(*head) = newNode;
}
// Function to display contents of the doubly linked list
void displayDlList(Node* head)
{
Node* last_node;
cout << "\n\nTraversal in Forward direction:\n";
while (head != NULL) {
cout << " " << head->data << " "; // Displaying data in forward direction
last_node = head;
head = head->next;
}
cout << "\n\nTraversal in Reverse direction:\n";
while (last_node != NULL) {
cout << " " << last_node->data << " "; // Displaying data in reverse direction
last_node = last_node->prev;
}
}
// Main program
int main() {
/* Start with the empty list */
struct Node* head = NULL; // Initializing the head of the linked list as NULL
append_data(&head, "Red"); // Appending "Red" at the front of the list
append_data(&head, "Green"); // Appending "Green" at the front of the list
append_data(&head, "White"); // Appending "White" at the front of the list
append_data(&head, "Orange"); // Appending "Orange" at the front of the list
cout<<"Doubly linked list is as follows: ";
displayDlList(head); // Displaying the doubly linked list
return 0; // Returning from the main function
}
Sample Output:
Doubly linked list is as follows: Traversal in Forward direction: Orange White Green Red Traversal in Reverse direction: Red Green White Orange
Flowchart:
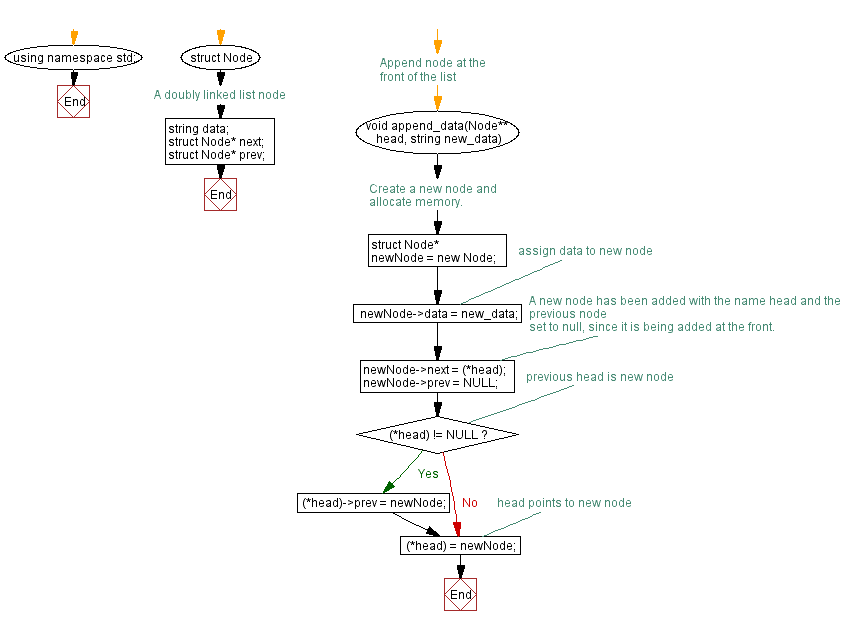
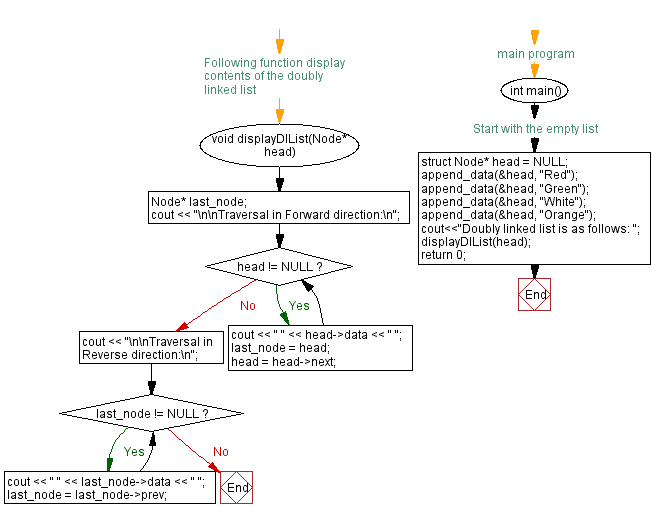
For more Practice: Solve these Related Problems:
- Write a C++ program to build a doubly linked list from user input and then display the list in both forward and reverse order.
- Develop a C++ program that creates a doubly linked list and prints each node's data along with its previous and next pointers.
- Design a C++ program to construct a doubly linked list and display it in a formatted style with highlighted head and tail nodes.
- Implement a C++ program to create a doubly linked list and then traverse it in reverse without using recursion.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Kth node from the Middle towards Head of a Linked List.
Next C++ Exercise: Reverse doubly linked list.
What is the difficulty level of this exercise?