C++ Exercises: Count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n
10. Count Total Occurrences of Digit 1 Up to n
Write a C++ program to count the total number of digits 1 appearing in all positive integers less than or equal to a given integer n.
Input n = 12,
Return 5, because digit 1 occurred 5 times in the following numbers: 1, 10, 11, 12.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count the number of times digit 1 appears in numbers up to a given limit
int count_DigitOne(int num) {
int m = 0, k = 0, result = 0, base = 1; // Initialize variables for calculations
// Loop through each digit of the number
while (num > 0) {
k = num % 10; // Extract the rightmost digit
num = num / 10; // Remove the rightmost digit
// Determine the count of digit 1 in the current position
if (k > 1) {
result += (num + 1) * base;
} else if (k < 1) {
result += num * base;
} else {
result += num * base + m + 1;
}
m += k * base; // Update the value of m for the next iteration
base *= 10; // Move to the next position (tens, hundreds, etc.)
}
return result; // Return the total count of digit 1 in the given number
}
int main(void) {
// Test cases to count the occurrences of digit 1 in numbers up to a certain limit
int n = 6;
cout << "\nTotal number of digit 1 appearing in " << n << " (less than or equal) is " << count_DigitOne(n) << endl;
n = 15;
cout << "\nTotal number of digit 1 appearing in " << n << " (less than or equal) is " << count_DigitOne(n) << endl;
n = 100;
cout << "\nTotal number of digit 1 appearing in " << n << " (less than or equal) is " << count_DigitOne(n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Total number of digit 1 appearing in 6 (less than or equal) is 1 Total number of digit 1 appearing in 15 (less than or equal) is 8 Total number of digit 1 appearing in 100 (less than or equal) is 21
Flowchart:
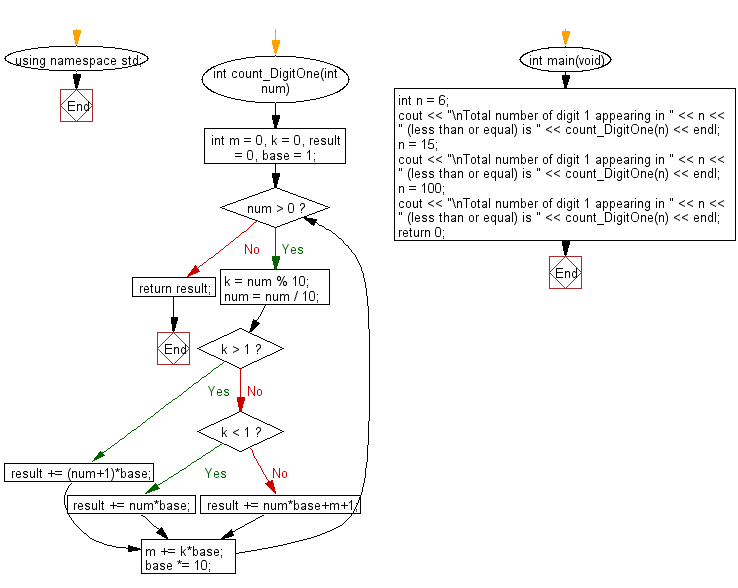
For more Practice: Solve these Related Problems:
- Write a C++ program to count how many times the digit 1 appears in all numbers from 1 to n by iterating through each number's digits.
- Write a C++ program that computes the frequency of the digit 1 in a range using mathematical formulas to avoid iterating through every number.
- Write a C++ program to recursively count occurrences of digit 1 in all numbers up to n and output the total count.
- Write a C++ program to determine the total count of digit 1 in numbers from 1 to n by analyzing each digit position separately.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the number of trailing zeroes in a given factorial.
Next: Write a C++ programming to add repeatedly all digits of a given non-negative number until the result has only one digit.
What is the difficulty level of this exercise?