C++ Exercises: Get the maximum product from a given integer after breaking the integer into the sum of at least two positive integers
Write a C++ program to get the maximum product of a given integer after breaking the integer into the sum of at least two positive integers.
Example-1:
Input: 12
Output: 81
Explanation: 12 = 3 + 3 + 3 + 3, 3 x 3 × 3 × 3 = 81.
Example-2:
Input: 7
Output: 12
Explanation: 7 = 3 + 2 + 2, 3 x 2 x 2 = 12.
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to compute the maximum product of an integer after breaking it
int integer_Break(int n) {
// Special cases for n = 2 and n = 3
if (n == 2) {
return 1;
} else if (n == 3) {
return 2;
} else if (n % 3 == 0) { // If n is divisible by 3
return (int)pow(3, n / 3); // Return 3 raised to the power of n/3
} else if (n % 3 == 1) { // If the remainder of n divided by 3 is 1
return 2 * 2 * (int)pow(3, (n - 4) / 3); // Return 2*2*3^(n-4)/3
} else { // For cases where the remainder of n divided by 3 is 2
return 2 * (int)pow(3, n / 3); // Return 2*3^(n/3)
}
}
int main() {
// Testing the integer_Break function for different values of n
int n = 7;
cout << "\nMaximum product of " << n << " after breaking the integer is " << integer_Break(n) << endl;
n = 9;
cout << "\nMaximum product of " << n << " after breaking the integer is " << integer_Break(n) << endl;
n = 12;
cout << "\nMaximum product of " << n << " after breaking the integer is " << integer_Break(n) << endl;
return 0;
}
Sample Output:
Maximum product of 7 after breaking the integer is 12 Maximum product of 9 after breaking the integer is 27 Maximum product of 12 after breaking the integer is 81
Flowchart:
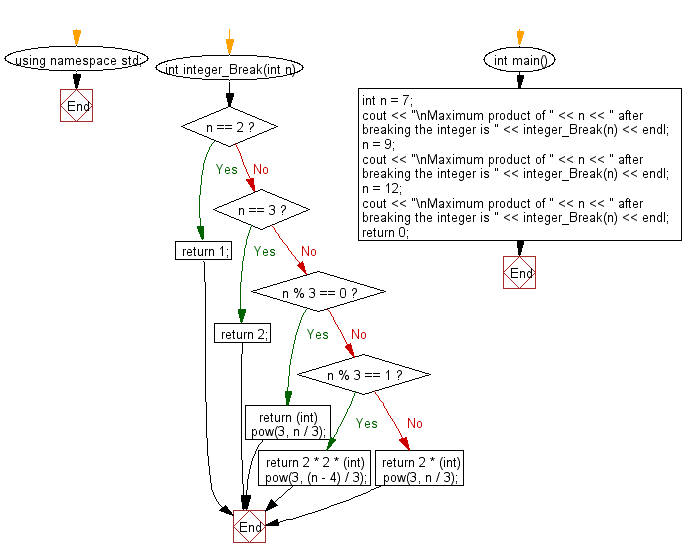
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to calculate the number of 1's in their binary representation and return them as an array.
Next: Write a C++ programming to find the nth digit of number 1 to n?.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics