C++ Exercises: Convert a given integer to a roman numeral
18. Integer to Roman Numeral Conversion
Write a C++ program to convert a given integer to a Roman numeral.
From Wikipedia:
Roman numerals are a numeral system that originated in ancient Rome and remained the usual way of writing numbers throughout Europe well into the Late Middle Ages. Numbers in this system are represented by combinations of letters from the Latin alphabet. Modern usage employs seven symbols, each with a fixed integer value:[1]
Sample Input: n = 7
Sample Output: Roman VII
Sample Input: n = 19
Sample Output: Roman XIX
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to convert an integer to its Roman numeral representation
string integer_to_Roman(int n) {
// Arrays holding Roman numeral symbols and their respective values
string str_romans[] = {"M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"};
int values[] = {1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1};
// Initialize an empty string to hold the resulting Roman numeral
string result = "";
// Loop through the values and corresponding Roman symbols
for (auto i = 0; i < 13; ++i)
{
// While the current number is greater than or equal to the value
while(n - values[i] >= 0)
{
// Append the Roman symbol to the result and subtract the value from the number
result += str_romans[i];
n -= values[i];
}
}
// Return the resulting Roman numeral
return result;
}
// Main function to test the integer_to_Roman function
int main()
{
// Test cases to convert integers to Roman numerals using the integer_to_Roman function
int n = 7;
cout << "Integer " << n << " : Roman " << integer_to_Roman(7) << endl;
n = 19;
cout << "Integer " << n << " : Roman " << integer_to_Roman(19) << endl;
n = 789;
cout << "Integer " << n << " : Roman " << integer_to_Roman(789) << endl;
n = 1099;
cout << "Integer " << n << " : Roman " << integer_to_Roman(1099) << endl;
n = 23456;
cout << "Integer " << n << " : Roman " << integer_to_Roman(23456) << endl;
return 0;
}
Sample Output:
Integer 7 : Roman VII Integer 19 : Roman XIX Integer 789 : Roman DCCLXXXIX Integer 1099 : Roman MXCIX Integer 23456 : Roman MMMMMMMMMMMMMMMMMMMMMMMCDLVI
Flowchart:
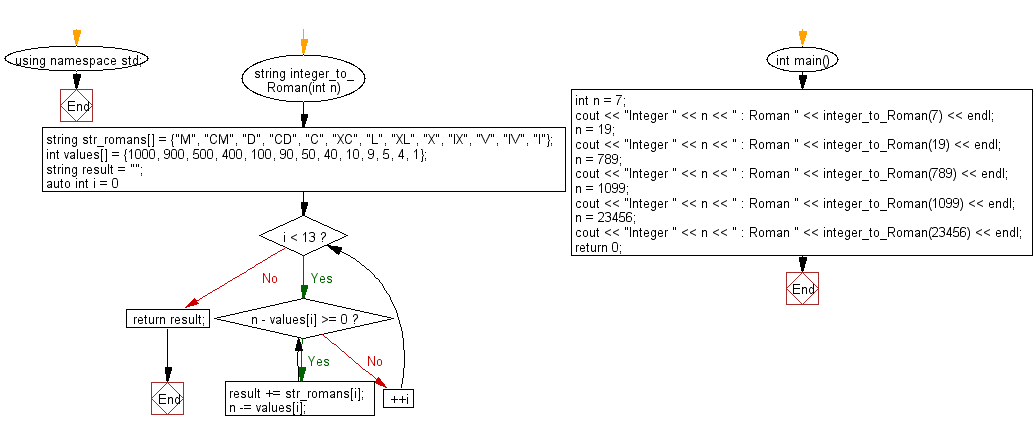
For more Practice: Solve these Related Problems:
- Write a C++ program to convert an integer to its Roman numeral representation using a greedy algorithm.
- Write a C++ program that reads an integer and constructs the corresponding Roman numeral by processing each digit.
- Write a C++ program to implement a function that maps integer values to Roman symbols and concatenates them in proper order.
- Write a C++ program to convert numbers to Roman numerals and handle edge cases like 4, 9, 40, 90, etc., correctly.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Next: Write a C++ program to convert a given roman numeral to a integer.
What is the difficulty level of this exercise?