C++ Exercises: Count all the numbers with unique digits in a range
29. Count Numbers with Distinct Digits of Length y
Write a C++ program that takes a number (n) and counts all numbers with distinct digits of length y within a specified range.
Range: 0 <= y < 10n
Example:
When n = 1, numbers with unique digits (10) between 0 and 9 are 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
When n = 2, numbers with unique digits (91) between 0 and 100 are 0,1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 13, 14, 15 …..99 except 11, 22, 33, 44, 55, 66, 77, 88 and 99.
Test Data:
1) -> 10
(2) -> 91
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to count the number of unique digit numbers given 'n' digits
int count_Unique_Digits_numbers(int n) {
// If 'n' is 0, there's only one number (0 itself)
if (n == 0) {
return 1;
}
int ctr = 10; // Initialize the counter to 10, considering single-digit numbers
for (int k = 2, fk = 9; k <= n; ++k) {
fk *= 10 - (k - 1); // Calculate the count of unique digits for 'k' digits and update 'fk'
ctr += fk; // Add the count of unique digits for 'k' digits to the total counter
}
return ctr; // Return the total count of unique digit numbers for 'n' digits
}
int main()
{
int n = 1;
// Display the number of unique digit numbers for 'n' digits
cout << "\nn = " << n << ", Number of unique digits: " << count_Unique_Digits_numbers(n) << endl;
n = 2;
// Display the number of unique digit numbers for 'n' digits
cout << "\nn = " << n << ", Number of unique digits: " << count_Unique_Digits_numbers(n) << endl;
return 0;
}
Sample Output:
n = 1, Number of unique digits: 10 n = 2, Number of unique digits: 91
Flowchart:
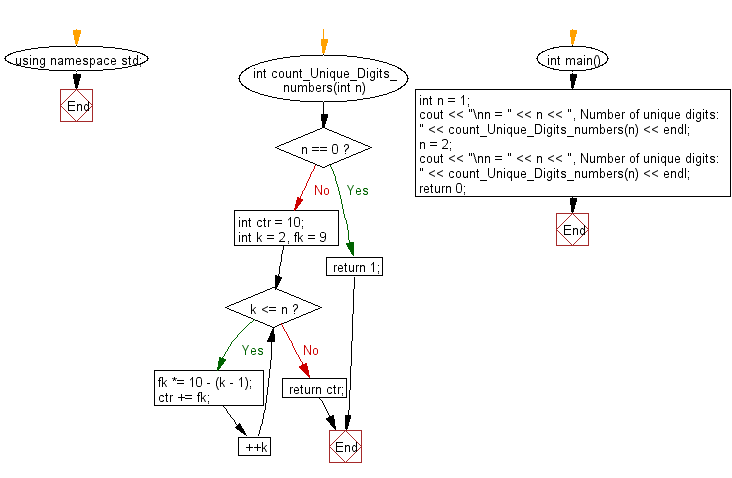
For more Practice: Solve these Related Problems:
- Write a C++ program to count all numbers with distinct digits of a given length using combinatorial calculations.
- Write a C++ program that generates numbers with distinct digits and counts them by iterating over possible digit combinations.
- Write a C++ program to compute the total count of distinct-digit numbers for a specified digit length using recursion.
- Write a C++ program to calculate the number of distinct-digit numbers in the range 0 to 10^n using permutation formulas.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to break a given integer in at least two parts (positive integers) to maximize the product of those integers.
Next: Write a C++ program to check whether a given positive integer is a perfect square or not.
What is the difficulty level of this exercise?