C++ Exercises: Divide two integers without using multiplication, division and mod operator
C++ Math: Exercise-4 with Solution
Write a C++ program to divide two integers (dividend and divisor) without using the multiplication, division and mod operators.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to perform integer division without using the division operator
int divide_result(int dividend_num, int divisor_num) {
// Handling special case where dividend is INT_MIN and divisor is -1 to prevent integer overflow
if (dividend_num == INT_MIN && divisor_num == -1) {
return INT_MAX;
}
// Checking if the result will be negative or positive
bool minus = ((dividend_num > 0) ^ (divisor_num > 0));
// Using long long to handle absolute values of numbers without causing overflow
long long num = labs(dividend_num); // Absolute value of dividend
long long div = labs(divisor_num); // Absolute value of divisor
int ans = 0; // Result of division
// Iteratively subtract divisor from dividend to find the quotient
while (num >= div) {
long long temp = div;
long long mul = 1;
// Doubling the divisor (left shift) to find the nearest multiple of divisor less than or equal to dividend
while (num >= (temp << 1)) {
mul <<= 1;
temp <<= 1;
}
ans += mul; // Increment the quotient by the multiple found
num -= temp; // Subtract the multiple from the dividend
}
return minus ? -ans : ans; // Return the final result with sign
}
int main(void) {
// Test cases for integer division and displaying results
int dividend_num = 7;
int divisor_num = 2;
cout << "\nDividend " << dividend_num << " Divisor " << divisor_num << endl;
cout << "Result: " << divide_result(dividend_num, divisor_num) << endl;
dividend_num = -17;
divisor_num = 5;
cout << "\nDividend " << dividend_num << " Divisor " << divisor_num << endl;
cout << "Result: " << divide_result(dividend_num, divisor_num) << endl;
dividend_num = 35;
divisor_num = 7;
cout << "\nDividend " << dividend_num << " Divisor " << divisor_num << endl;
cout << "Result: " << divide_result(dividend_num, divisor_num) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Dividend 7 Divisor 2 Result: 3 Dividend -17 Divisor 5 Result: -3 Dividend 35 Divisor 7 Result: 5
Flowchart:
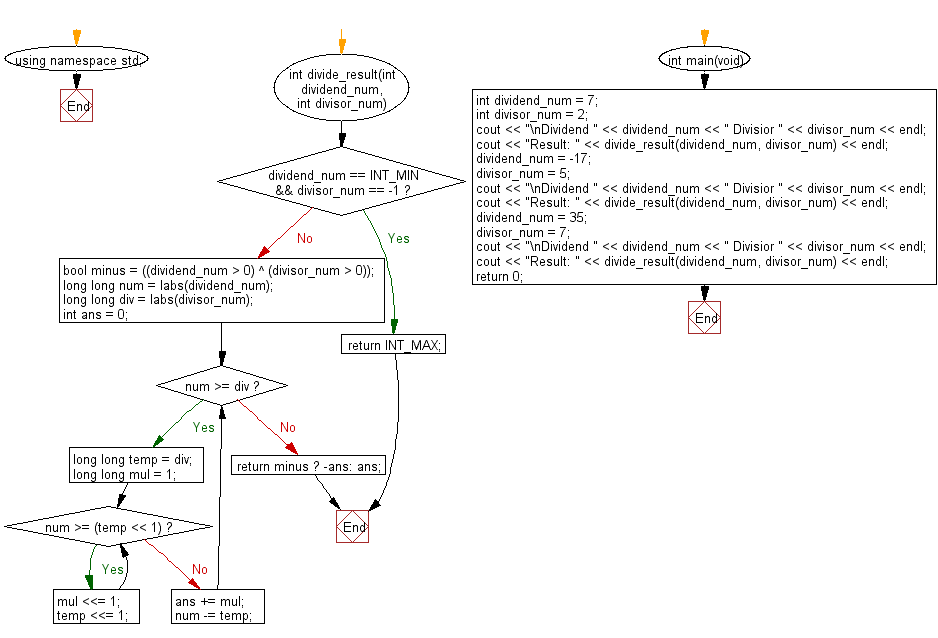
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to reverse the digits of a given integer.
Next: Write a C++ program to calculate x raised to the power n (xn).
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/math/cpp-math-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics