C++ Exercises: Check two numbers are Amicable numbers or not
C++ Numbers: Exercise-28 with Solution
Write a C++ program to check two numbers are Amicable numbers or not.
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard C++ libraries
using namespace std; // Using the standard namespace
int ProDivSum(int n) // Function to calculate the sum of proper divisors of a number 'n'
{
int sum = 1; // Initialize sum with 1 (as 1 is a divisor for all numbers)
for (int i = 2; i <= sqrt(n); i++) // Loop through numbers from 2 to square root of 'n'
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
sum += i; // Add 'i' to the sum
// If 'n' is divisible by 'i' but not equal to 'i', add the corresponding divisor to the sum
if (n / i != i)
sum += n / i;
}
}
return sum; // Return the sum of proper divisors
}
bool chkAmicable(int a, int b) // Function to check if two numbers 'a' and 'b' are amicable pairs
{
return (ProDivSum(a) == b && ProDivSum(b) == a); // Return true if the sum of proper divisors of 'a' equals 'b' and vice versa
}
int main()
{
int n, i, j, ctr, nm1, nm2; // Declare integer variables
cout << "\n\n Check whether two numbers are Amicable pairs or not: \n"; // Display a message
cout << "\n Sample: (220, 284), (1184, 1210), (2620, 2924).. \n";
cout << " --------------------------------------------------------\n";
cout << " Input the 1st number : ";
cin >> nm1; // Input the first number
cout << " Input the 2nd number : ";
cin >> nm2; // Input the second number
if (chkAmicable(nm1, nm2)) // Check if the input numbers are amicable pairs
cout << " The given numbers are an Amicable pair." << endl; // Display if they are amicable pairs
else
cout << " The given numbers are not an Amicable pair." << endl; // Display if they are not amicable pairs
return 0; // Exit the program
}
Sample Output:
Check whether two numbers are Amicable pairs or not: Sample: (220, 284), (1184, 1210), (2620, 2924).. -------------------------------------------------------- Input the 1st number : 220 Input the 2nd number : 284 The given numbers are an Amicable pair.
Flowchart:
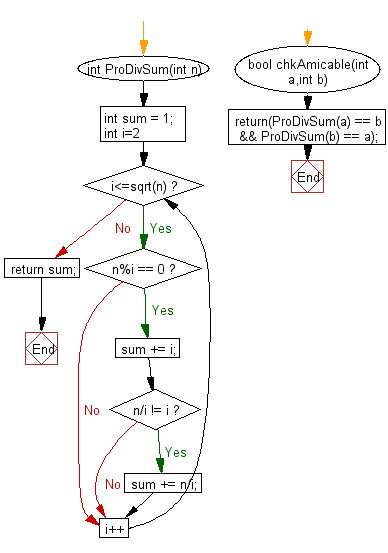
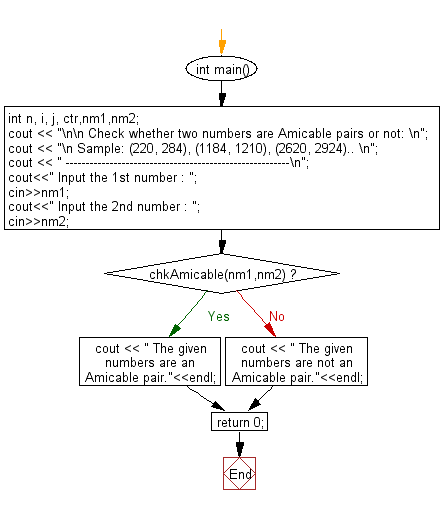
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Duck Numbers between 1 to 500.
Next: Write a program in C++ to count the Amicable pairs in an array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics