C++ Exercises: Check if a number is Mersenne number or not
35. Mersenne Number Check
Write a C++ program to check if a number is a Mersenne number or not.
Sample Solution:
C++ Code :
# include <iostream> // Including input-output stream library
# include <math.h> // Including math library for mathematical functions
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int n, p, ans, i, n1; // Declaring variables for storing input, power, answer, and loop control
double result; // Declaring a variable for storing the result of mathematical operations
cout << "\n\n Check whether a given number is a Mersenne number or not:\n"; // Display purpose
cout << "------------------------------------------------------------\n"; // Display purpose
cout << " Input a number: "; // Prompting user for input
cin >> n; // Taking user input
n1 = n + 1; // Calculating n+1 for further comparison
p = 0; // Initializing 'p' variable to store powers of 2
ans = 0; // Initializing 'ans' variable to determine if the number is Mersenne or not
for (i = 0;; i++) // Start of an infinite loop to generate powers of 2
{
p = (int)pow(2, i); // Calculating powers of 2 using the 'pow' function
if (p > n1) // If the calculated power exceeds the modified number 'n1', exit the loop
{
break;
}
else if (p == n1) // If the calculated power equals the modified number 'n1', it's a Mersenne number
{
cout << " " << n << " is a Mersenne number." << endl; // Displaying the result
ans = 1; // Setting the 'ans' variable to indicate the number is a Mersenne number
}
}
if (ans == 0) // If 'ans' is still 0, it means the number is not a Mersenne number
{
cout << " " << n << " is not a Mersenne number." << endl; // Displaying the result
}
}
Sample Output:
Check whether a given number is Mersenne number or not: ------------------------------------------------------------ Input a number: 31 31 is a Mersenne number.
Flowchart:
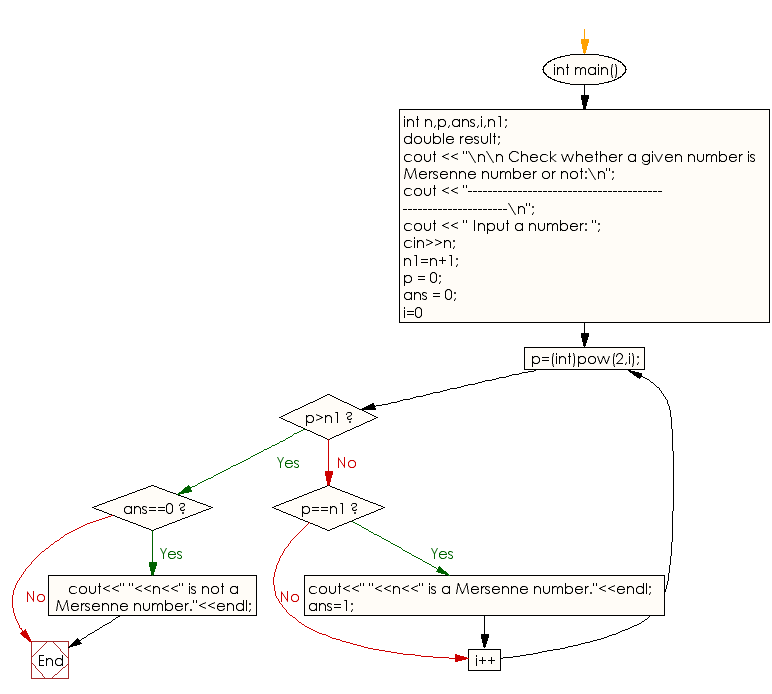
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is a Mersenne number by checking if it is one less than a power of two.
- Write a C++ program to verify Mersenne numbers using bit manipulation to test power-of-two conditions.
- Write a C++ program to check for Mersenne numbers by computing logarithms and validating integer results.
- Write a C++ program to determine Mersenne number status with custom functions for efficient bitwise operations.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find any number between 1 and n that can be expressed as the sum of two cubes in two (or more) different ways.
Next: Write a program in C++ to generate Mersenne primes within a range of numbers.
What is the difficulty level of this exercise?