C++ Exercises: Check if a number is Keith or not
Find Strong Numbers Within a Range
Write a C++ program to check if a number is Keith or not.
Sample Output:
Input a number : 742
The given number is a Keith Number.
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Including the complete set of standard C++ libraries
using namespace std; // Using the standard namespace
int lenCount(int nm) // Function to count the number of digits in a number
{
int ctr = 0; // Counter initialized to count the digits
while (nm > 0) // Loop to count digits
{
nm = nm / 10; // Remove the last digit
ctr++; // Increment the counter for each digit removed
}
return ctr; // Return the count of digits
}
int main() // Main function
{
int num1 = 0, arr1[10], num2 = 0, flg = 0, i = 0, sum = 0; // Declaration of integer variables
cout << "\n\n Check whether a number is Keith or not: \n"; // Displaying purpose
cout << " Sample Keith numbers: 197, 742, 1104, 1537, 2208, 2580, 3684, 4788, 7385..\n"; // Displaying purpose
cout << " -----------------------------------------------------------------------\n"; // Displaying purpose
cout << " Input a number : "; // Prompting user for input
cin >> num1; // Taking user input
num2 = num1; // Storing the original number
// Loop to store individual digits of the number into an array in reverse order
for (i = lenCount(num2) - 1; i >= 0; i--)
{
arr1[i] = num1 % 10; // Extract the last digit
num1 /= 10; // Remove the last digit
}
// Loop to check if the number is a Keith number
while (flg == 0)
{
// Calculate the sum of the digits in the array
for (i = 0; i < lenCount(num2); i++)
sum += arr1[i];
// If the sum matches the original number, it's a Keith number
if (sum == num2)
{
cout << " The given number is a Keith Number.\n"; // Displaying output
flg = 1; // Set the flag to terminate the loop
}
// If the sum exceeds the original number, it's not a Keith number
if (sum > num2)
{
cout << " The given number is not a Keith number.\n"; // Displaying output
flg = 1; // Set the flag to terminate the loop
}
// Reconstruct the array by shifting elements and adding the new sum
for (i = 0; i < lenCount(num2); i++)
{
if (i != lenCount(num2) - 1)
arr1[i] = arr1[i + 1]; // Shift elements in the array
else
arr1[i] = sum; // Store the new sum at the last index of the array
}
sum = 0; // Reset the sum for the next iteration
}
}
Sample Output:
Check whether a number is Keith or not: Sample Keith numbers: 197, 742, 1104, 1537, 2208, 2580, 3684, 4788, 7385.. ----------------------------------------------------------------------- Input a number : 742 The given number is a Keith Number.
Flowchart:
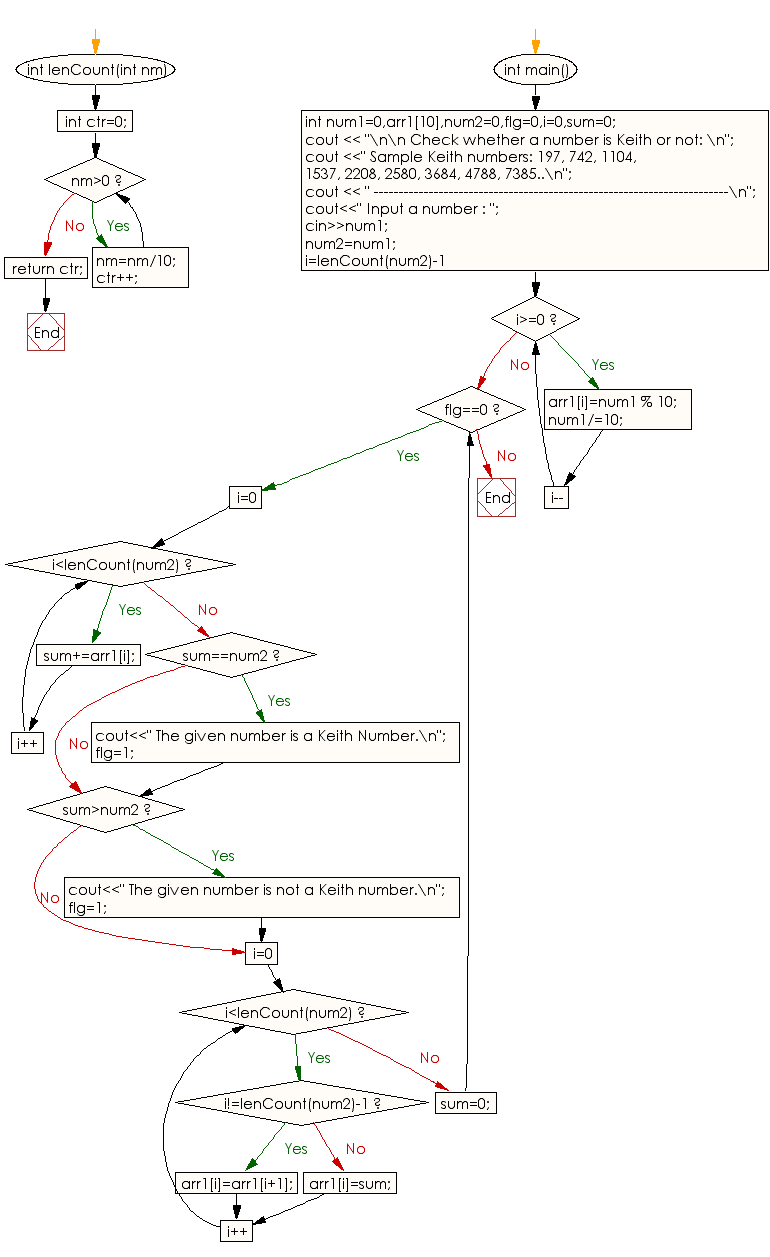
For more Practice: Solve these Related Problems:
- Write a C++ program to list all strong numbers in a given range by comparing the sum of factorials of digits.
- Write a C++ program to identify strong numbers between two limits using efficient factorial calculation.
- Write a C++ program to generate strong numbers within a range with inline functions for factorial computation.
- Write a C++ program to display strong numbers and count them using STL algorithms for processing digit factorials.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to print the first 20 numbers of the Pell series.
Next: Write a program in C++ to check if a number is Keith or not.
What is the difficulty level of this exercise?