C++ Queue Exercises: Implement a queue using an array
1. Queue Using Array with Enqueue/Dequeue and Status Check
Write a C++ program to implement a queue using an array with enqueue and dequeue operations. Find the top element of the stack and check if the stack is empty, full or not.
Note: Putting items in the queue is called enqueue, and removing items from the queue is called dequeue.
Sample Solution:
C Code:
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
class Queue {
private:
int front; // Front index of the queue
int rear; // Rear index of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initialize front index to -1
rear = -1; // Initialize rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error message if queue is full
return;
}
if (isEmpty()) {
front = 0; // If the queue is empty, update front to 0
rear = 0; // If the queue is empty, update rear to 0
} else {
rear++; // Increment rear index
}
arr[rear] = x; // Insert the element at the rear index
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
if (front == rear) {
front = -1; // If the queue has only one element, update front to -1
rear = -1; // If the queue has only one element, update rear to -1
} else {
front++; // Increment front index
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error message if queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nIs the Queue is empty? " << q.isEmpty() << endl;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(5);
cout << "\nIs the Queue full? " << q.isFull() << endl;
cout << "\nIs the Queue is empty? " << q.isEmpty() << endl;
q.display();
cout << "\nFront element is " << q.peek() << endl;
cout << "\nRemove two elements from the Queue" << endl;
q.dequeue();
q.dequeue();
q.display();
cout << "\nFront element is " << q.peek() << endl;
return 0;
}
Sample Output:
Initialize a Queue. Is the Queue is empty? 1 Insert some elements into the queue: Is the Queue full? 0 Is the Queue is empty? 0 Queue elements are: 1 2 3 4 5 Front element is 1 Remove two elements from the Queue Queue elements are: 3 4 5 Front element is 3
Flowchart:
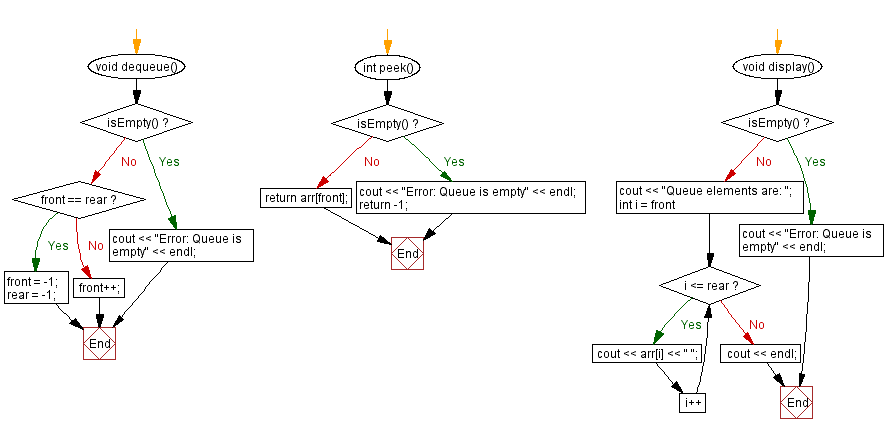
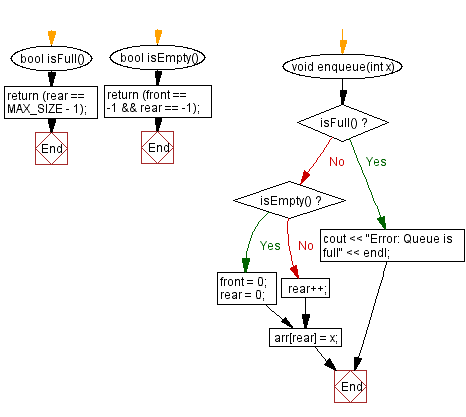
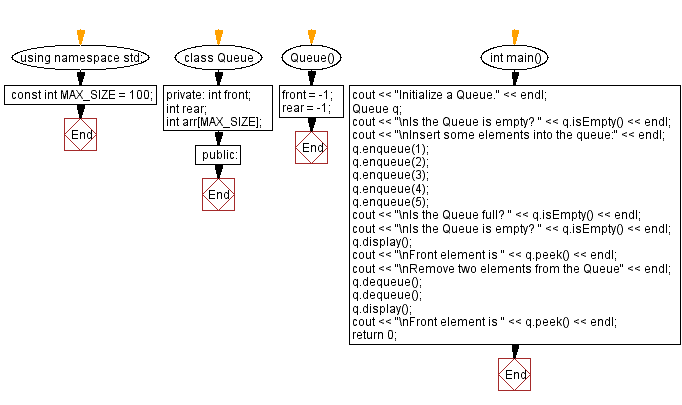
For more Practice: Solve these Related Problems:
- Write a C++ program to implement a circular queue using an array that supports enqueue, dequeue, and full/empty checks.
- Write a C++ program to simulate a priority queue using an array where insertion is based on a priority value.
- Write a C++ program that implements a queue using an array and periodically reports its occupancy status during operations.
- Write a C++ program to design a fixed-size queue using an array and trigger custom alerts when the queue is either full or empty.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: C++ Queue Exercises Home.
Next C++ Exercise: Reverse the elements of a queue.
What is the difficulty level of this exercise?