C++ Queue Exercises: Remove a given element from a queue
C++ Queue: Exercise-13 with Solution
Write a C++ program to remove a given element from a queue.
Sample Solution:
C Code:
#include <iostream>
#include <map>
#include <cmath>
using namespace std;
const int MAX_SIZE = 100;
class Queue {
private:
int front; // Holds the front index of the queue
int rear; // Holds the rear index of the queue
int arr[MAX_SIZE]; // Array to hold queue elements
public:
Queue() {
front = -1; // Initialize front index to -1
rear = -1; // Initialize rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Checks if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Checks if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Displays error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
} else {
rear++;
}
arr[rear] = x; // Adds an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
} else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return -1;
}
return arr[front]; // Returns the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Displays error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Displays all elements in the queue
}
cout << endl;
}
void remove_element(Queue & q, int x) {
Queue temp; // Temporary queue to hold elements while removing the specified element
bool found = false; // Flag to indicate if the element is found
while (!q.isEmpty()) {
int curr = q.peek();
q.dequeue();
if (curr == x) {
found = true; // Element to be removed is found
continue; // Skip adding this element to the temporary queue
}
temp.enqueue(curr); // Add elements other than the one to be removed to the temporary queue
}
if (!found) {
cout << "Error: Element not found in queue" << endl; // Element not found in the queue
return;
}
while (!temp.isEmpty()) {
int curr = temp.peek();
temp.dequeue();
q.enqueue(curr); // Re-add elements back to the original queue from the temporary queue
}
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q;
cout << "\nInsert some elements into the queue:" << endl;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(5);
q.enqueue(6);
q.display();
cout << "\nRemove 5 from the said queue:" << endl;
q.remove_element(q, 5);
q.display();
cout << "\nRemove 1 from the said queue:" << endl;
q.remove_element(q, 1);
q.display();
cout << "\nRemove 6 from the said queue:" << endl;
q.remove_element(q, 6);
q.display();
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue elements are: 1 2 3 4 5 6 Remove 5 from the said queue: Queue elements are: 1 2 3 4 6 Remove 1 from the said queue: Queue elements are: 2 3 4 6 Remove 6 from the said queue: Queue elements are: 2 3 4
Flowchart:
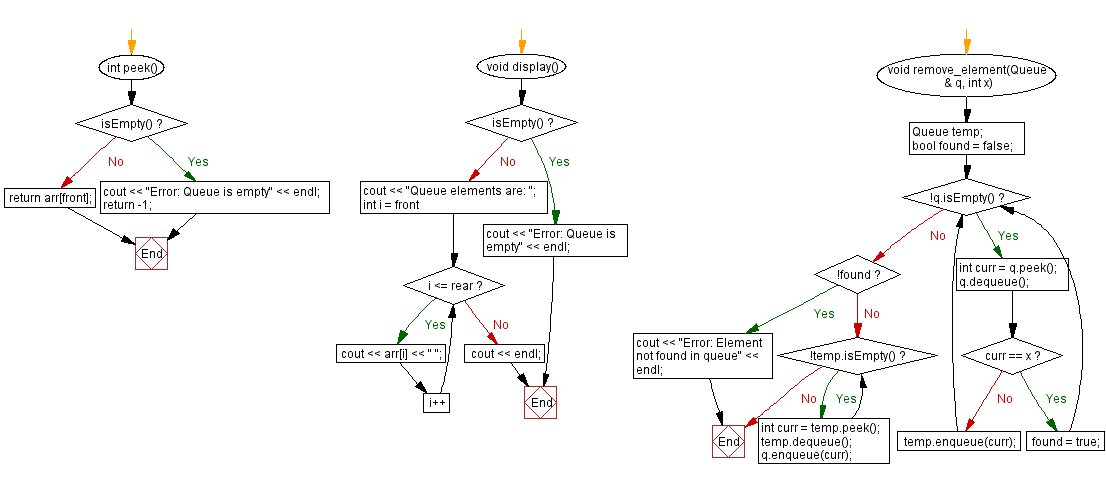
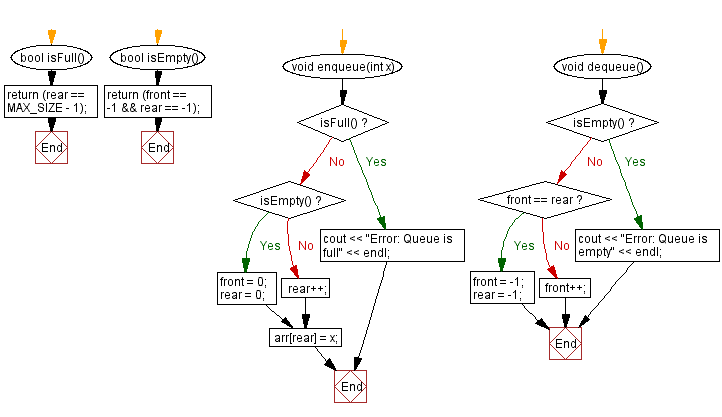
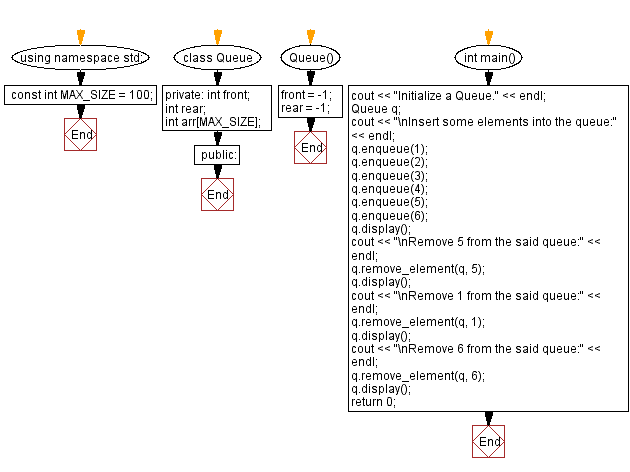
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find the second lowest element of a queue.
Next C++ Exercise: Remove all the elements from a queue.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/queue/cpp-queue-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics