C++ Queue Exercises: Concatenate two queues
C++ Queue: Exercise-19 with Solution
Write a C++ program to concatenate two queues.
Sample Solution:
C Code:
#include <iostream> // Including necessary library for input and output operations
using namespace std;
const int MAX_SIZE = 100; // Maximum size for the queue
class Queue {
private:
int front; // Front of the queue
int rear; // Rear of the queue
int arr[MAX_SIZE]; // Array to store queue elements
public:
Queue() {
front = -1; // Initializing front index to -1
rear = -1; // Initializing rear index to -1
}
bool isFull() {
return (rear == MAX_SIZE - 1); // Check if the queue is full
}
bool isEmpty() {
return (front == -1 && rear == -1); // Check if the queue is empty
}
void enqueue(int x) {
if (isFull()) {
cout << "Error: Queue is full" << endl; // Display error if the queue is full
return;
}
if (isEmpty()) {
front = 0;
rear = 0;
}
else {
rear++;
}
arr[rear] = x; // Add an element to the rear of the queue
}
void dequeue() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
if (front == rear) {
front = -1;
rear = -1;
}
else {
front++;
}
}
int peek() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return -1;
}
return arr[front]; // Return the element at the front of the queue
}
void display() {
if (isEmpty()) {
cout << "Error: Queue is empty" << endl; // Display error if the queue is empty
return;
}
cout << "Queue elements are: ";
for (int i = front; i <= rear; i++) {
cout << arr[i] << " "; // Display all elements in the queue
}
cout << endl;
}
// Function to concatenate two queues
void concatenate(Queue& q1, Queue& q2) {
while (!q2.isEmpty()) {
q1.enqueue(q2.peek()); // Enqueue elements from q2 into q1
q2.dequeue(); // Dequeue elements from q2
}
}
};
int main() {
cout << "Initialize a Queue." << endl;
Queue q1, q2, result_queue;
cout << "\nInsert some elements into the queue:" << endl;
q1.enqueue(1);
q1.enqueue(2);
q1.enqueue(3);
q1.enqueue(4);
cout << "Queue-1" << endl;
q1.display(); // Display elements of queue 1
q2.enqueue(5);
q2.enqueue(6);
q2.enqueue(1);
cout << "Queue-2" << endl;
q2.display(); // Display elements of queue 2
q1.concatenate(q1, q2); // Concatenate queue 1 and queue 2
cout << "\nConcatenate said two queues:" << endl;
q1.display(); // Display concatenated queue
return 0;
}
Sample Output:
Initialize a Queue. Insert some elements into the queue: Queue-1 Queue elements are: 1 2 3 4 Queue-2 Queue elements are: 5 6 1 Concatenate said two queues: Queue elements are: 1 2 3 4 5 6 1
Flowchart:
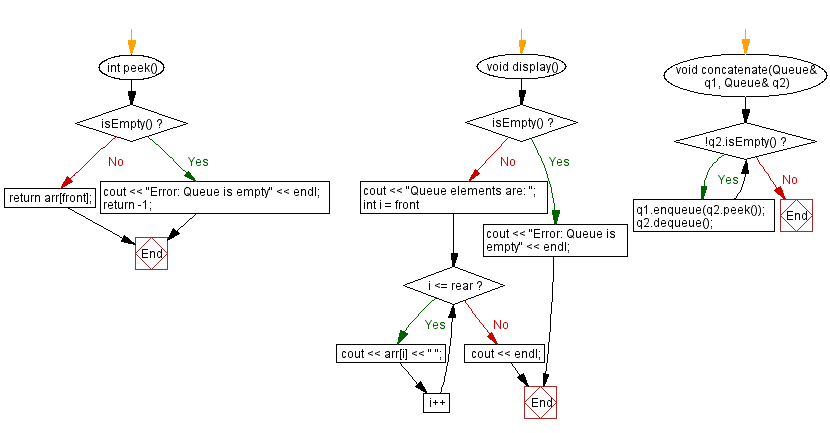
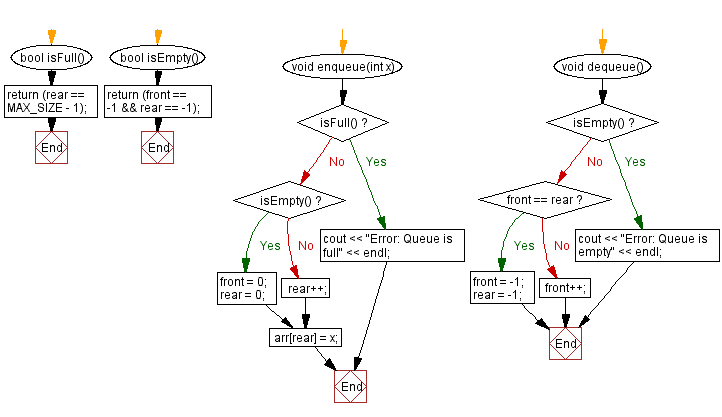
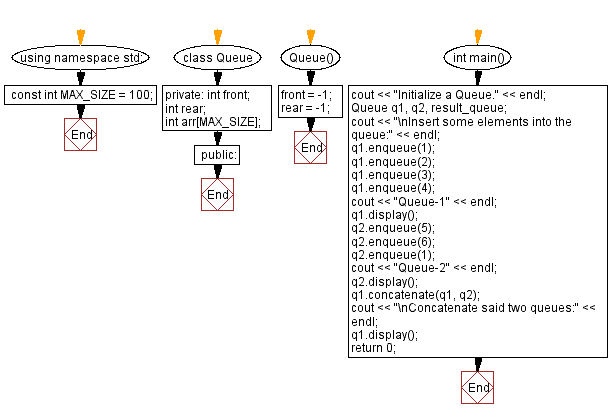
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove all elements greater than a number from a queue.
Next C++ Exercise: Copy one queue to another.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/queue/cpp-queue-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics