C++ Recursion: Calculating the sum of even and odd numbers in a range
14. Recursive Sum of Even and Odd Numbers in a Range
Write a C++ program to implement a recursive function to calculate the sum of even and odd numbers in a given range.
Sample Solution:
C Code:
// Recursive function to calculate the sum of even and odd numbers in a range
#include <iostream>
// Recursive function to calculate the sum of even and odd numbers within a given range
void calculate_even_odd_Sum(int start, int end, int & evenSum, int & oddSum) {
// Base case: If start becomes greater than end, stop the recursion
if (start > end)
return;
// Recursive case: add the current number to the appropriate sum
if (start % 2 == 0)
evenSum += start; // Add the current number to the even sum
else
oddSum += start; // Add the current number to the odd sum
// Recursively call the function with the next number in the range
calculate_even_odd_Sum(start + 1, end, evenSum, oddSum);
}
int main() {
int start, end;
std::cout << "Input the starting number: ";
std::cin >> start;
std::cout << "Input the ending number: ";
std::cin >> end;
int even_Sum = 0;
int odd_Sum = 0;
// Calculate the sum of even and odd numbers using recursion
calculate_even_odd_Sum(start, end, even_Sum, odd_Sum);
std::cout << "Sum of even numbers: " << even_Sum << std::endl;
std::cout << "Sum of odd numbers: " << odd_Sum << std::endl;
return 0;
}
Sample Output:
Input the starting number: 1 Input the ending number: 10 Sum of even numbers: 30 Sum of odd numbers: 25
Explanation:
In the above exercise,
- The "calculate_even_odd_Sum()" function takes the starting number (start), ending number (end), and two integer references (evenSum and oddSum) as parameters.
- It uses recursion to calculate the sum of even and odd numbers in the given range.
- The base case is when the start is greater than the end, in which case the function stops recursion.
- In the recursive case, the function checks if the start number is even or odd using the modulo operator (%).
- If it's even, it adds it to the even_Sum variable; otherwise, it adds it to the odd_Sum variable.
- Then, it recursively calls the function with the next number in the range (start + 1). The recursive calls continue until the base case is reached.
The "main()" function prompts the user to input the starting and ending numbers. It initializes the even_Sum and odd_Sum variables, calls the "calculate_even_odd_Sum()" function to calculate the sums using recursion, and then displays the sums of even and odd numbers on the console.
Flowchart:
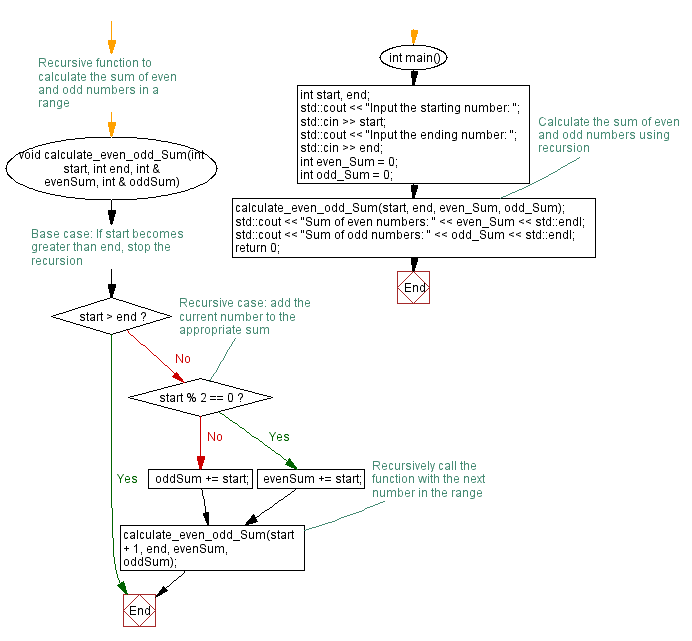
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively calculate the sum of even numbers and the sum of odd numbers within a given range.
- Write a C++ program that uses recursion to compute and print separately the sums of even and odd numbers between two integers.
- Write a C++ program to implement a recursive function that returns a pair containing the sum of evens and odds in a specified range.
- Write a C++ program that reads two integers and recursively computes the sum of even numbers and odd numbers, then outputs both totals.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Calculating product of two numbers without multiplication operator.
Next C++ Exercise: Checking if a binary tree is a binary search tree.
What is the difficulty level of this exercise?