C++ Selection sort Exercise: Sort a collection of integers using the Selection sort
14. Sort a Collection Using the Selection Sort Algorithm
Write a C++ program to sort a collection of integers using Selection sort.
Sample Solution:
C++ Code :
#include <algorithm>
#include <iterator>
#include <iostream>
// Selection sort implementation using ForwardIterator
template<typename ForwardIterator>
void selection_sort(ForwardIterator begin, ForwardIterator end) {
for (auto i = begin; i != end; ++i) {
// Swap the current element with the minimum element in the unsorted portion of the range
std::iter_swap(i, std::min_element(i, end));
}
}
// Main function
int main() {
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using selection sort
selection_sort(std::begin(a), std::end(a));
// Displaying the sorted numbers after selection sort
std::cout << "Sorted numbers:\n";
copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted numbers: -256 -5 0 3 44 55 125 214 695
Flowchart:
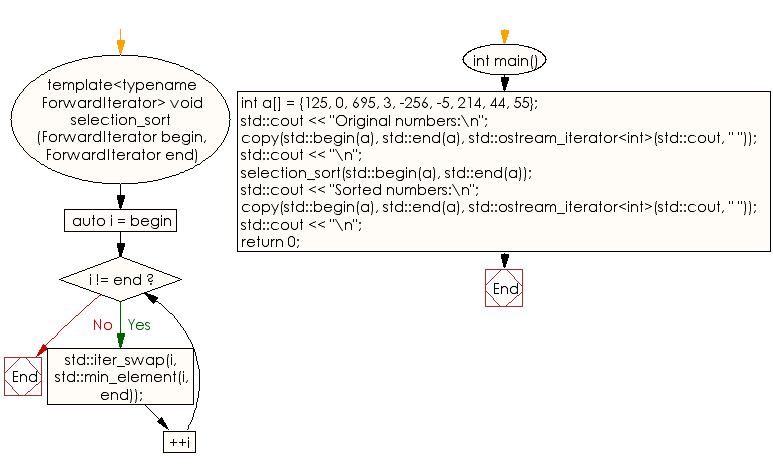
For more Practice: Solve these Related Problems:
- Write a C++ program to implement selection sort and print the index of the minimum element found in each pass.
- Write a C++ program to implement selection sort recursively and display the array state after each selection.
- Write a C++ program to implement selection sort and count the total number of comparisons performed.
- Write a C++ program to sort an array of custom objects using selection sort with overloaded comparison operators.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort a collection of integers using the Radix sort.
What is the difficulty level of this exercise?