C++ Stack Exercises: Find and remove the lowest element in a stack
C++ Stack: Exercise-11 with Solution
Write a C++ program to find and remove the lowest element in a stack.
Test Data:
Input some elements onto the stack:
Stack elements are: 5 2 4 7
Find and remove the lowest element 2 from the stack.
Stack elements are: 5 4 7
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
#define MAX_SIZE 15 // Maximum size of stack
class Stack {
private:
int top; // Index of top element
int arr[MAX_SIZE]; // Array to store elements
public:
Stack() {
top = -1; // Initialize top index to -1 (empty stack)
}
bool push(int x) {
if (isFull()) {
cout << "Stack overflow" << endl; // Display message if stack is full
return false; // Return false to indicate failure in pushing element
}
// Increment top index and add element to array
arr[++top] = x;
return true; // Return true to indicate successful element addition
}
int pop() {
if (isEmpty()) {
cout << "Stack underflow" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in popping element
}
// Return top element and decrement top index
return arr[top--];
}
int peek() {
if (isEmpty()) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in peeking element
}
// Return top element without modifying top index
return arr[top];
}
bool isEmpty() {
// Stack is empty if top index is -1
return (top < 0);
}
bool isFull() {
// Stack is full if top index is equal to MAX_SIZE - 1
return (top >= MAX_SIZE - 1);
}
void display() {
if (top < 0) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return;
}
cout << "\nStack elements are: ";
for (int i = top; i >= 0; i--)
cout << arr[i] << " "; // Display elements of the stack
cout << endl;
}
int find_and_remove_lowest() {
if (isEmpty()) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return -1; // Return -1 to indicate failure in finding the lowest element
}
int minElement = INT_MAX; // Initialize minElement with the largest possible integer value
Stack temp;
while (!isEmpty()) {
int element = pop();
if (element < minElement) {
minElement = element; // Update minElement if a smaller element is found
}
temp.push(element); // Push other elements onto the temporary stack
}
while (!temp.isEmpty()) {
int element = temp.pop();
if (element != minElement) { // Push all elements except for the minElement back onto the original stack
push(element);
}
}
return minElement; // Return the lowest element that was removed
}
};
int main() {
// Initialize the stack stk
Stack stk;
cout << "Input some elements onto the stack:";
stk.push(7);
stk.push(4);
stk.push(2);
stk.push(5);
stk.display();
int z = stk.find_and_remove_lowest();
cout << "Find and remove the lowest element " << z << " from the stack.";
stk.display();
cout << "\nInput two more elements";
stk.push(-1);
stk.push(2);
stk.display();
z = stk.find_and_remove_lowest();
cout << "Find and remove the lowest element " << z << " from the stack.";
stk.display();
cout << endl;
return 0;
}
Sample Output:
Input some elements onto the stack: Stack elements are: 5 2 4 7 Find and remove the lowest element 2 from the stack. Stack elements are: 5 4 7 Input two more elements Stack elements are: 2 -1 5 4 7 Find and remove the lowest element -1 from the stack. Stack elements are: 2 5 4 7
Flowchart:
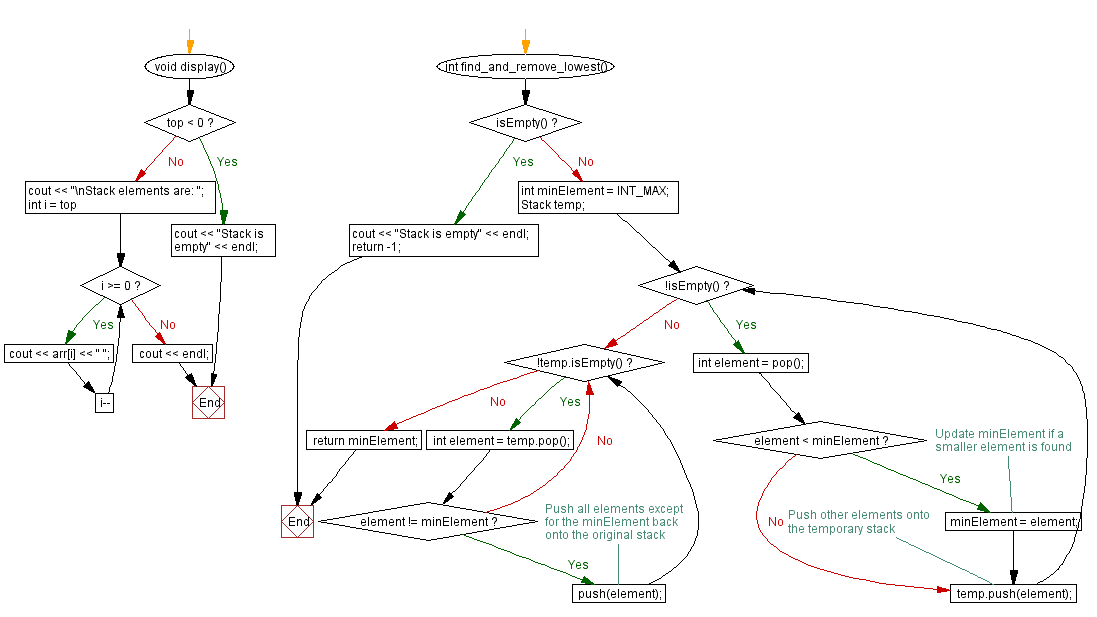
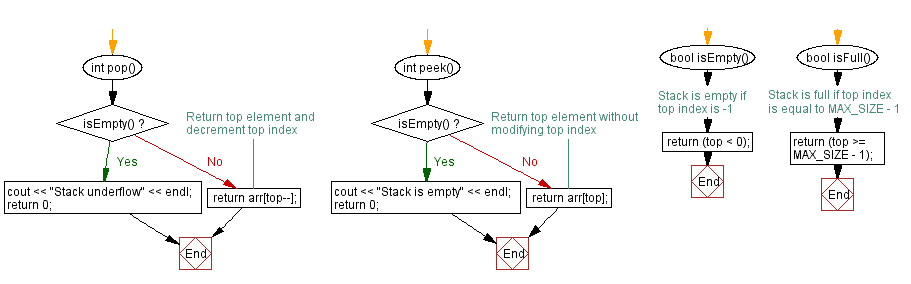
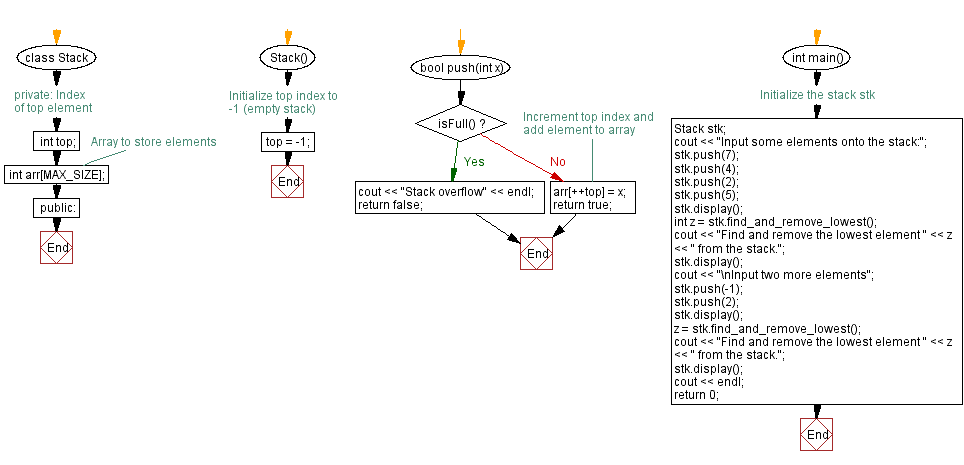
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Find and remove the largest element in a stack.
Next C++ Exercise: Remove duplicates from a stack using arrays.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics