C++ Stack Exercises: Find the maximum element in a stack (using an array)
C++ Stack: Exercise-6 with Solution
Write a C++ program to find the maximum element in a stack (using an array).
Test Data:
Input some elements onto the stack:
Stack elements: 0 1 5 2 4 7
Maximum value: 7
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
#define MAX_SIZE 15 // Maximum size of stack
class Stack {
private:
int top; // Index of top element
int arr[MAX_SIZE]; // Array to store elements
public:
Stack() {
top = -1; // Initialize top index to -1 (empty stack)
}
bool push(int x) {
if (isFull()) {
cout << "Stack overflow" << endl; // Display message if stack is full
return false; // Return false to indicate failure in pushing element
}
// Increment top index and add element to array
arr[++top] = x;
return true; // Return true to indicate successful element addition
}
int pop() {
if (isEmpty()) {
cout << "Stack underflow" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in popping element
}
// Return top element and decrement top index
return arr[top--];
}
int peek() {
if (isEmpty()) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return 0; // Return 0 to indicate failure in peeking element
}
// Return top element without modifying top index
return arr[top];
}
bool isEmpty() {
// Stack is empty if top index is -1
return (top < 0);
}
bool isFull() {
// Stack is full if top index is equal to MAX_SIZE - 1
return (top >= MAX_SIZE - 1);
}
void display() {
if (top < 0) {
cout << "Stack is empty" << endl; // Display message if stack is empty
return;
}
cout << "\nStack elements: ";
for (int i = top; i >= 0; i--)
cout << arr[i] << " "; // Display elements of the stack
cout << endl;
}
int max() {
if (isEmpty()) {
// Return the minimum possible integer if the stack is empty
return INT_MIN;
}
int maxVal = arr[0];
for (int i = 1; i <= top; i++) {
if (arr[i] > maxVal) {
// Update the maximum value if we find a larger element
maxVal = arr[i];
}
}
// Return the maximum value
return maxVal;
}
};
int main() {
// Initialize the stack stk
Stack stk;
cout << "Input some elements onto the stack:";
stk.push(7);
stk.push(4);
stk.push(2);
stk.push(5);
stk.push(1);
stk.push(0);
stk.display();
// Find the maximum value
int max_val = stk.max();
cout << "\nMaximum value: " << max_val << endl; // Display the maximum value
cout << "\nRemove two elements:";
stk.pop();
stk.pop();
stk.display();
cout << "\nInput two more elements";
stk.push(-1);
stk.push(10);
stk.display();
max_val = stk.max();
cout << "\nMaximum value: " << max_val << endl; // Display the maximum value
cout << endl;
return 0;
}
Sample Output:
Input some elements onto the stack: Stack elements: 0 1 5 2 4 7 Maximum value: 7 Remove two elements: Stack elements: 5 2 4 7 Input two more elements Stack elements: 10 -1 5 2 4 7 Maximum value: 10
Flowchart:
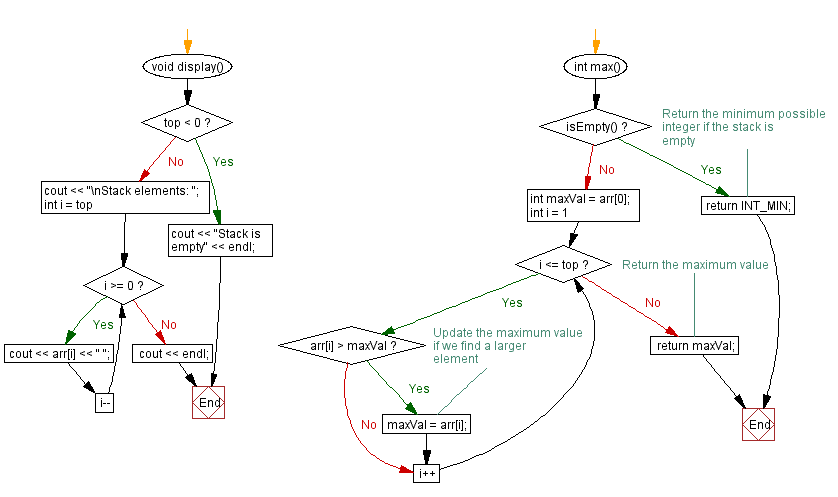
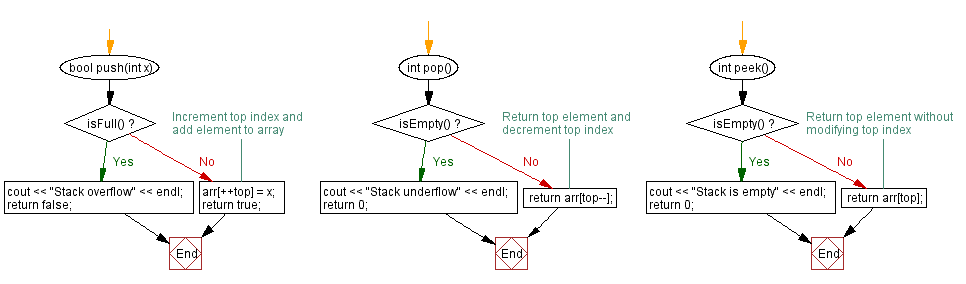
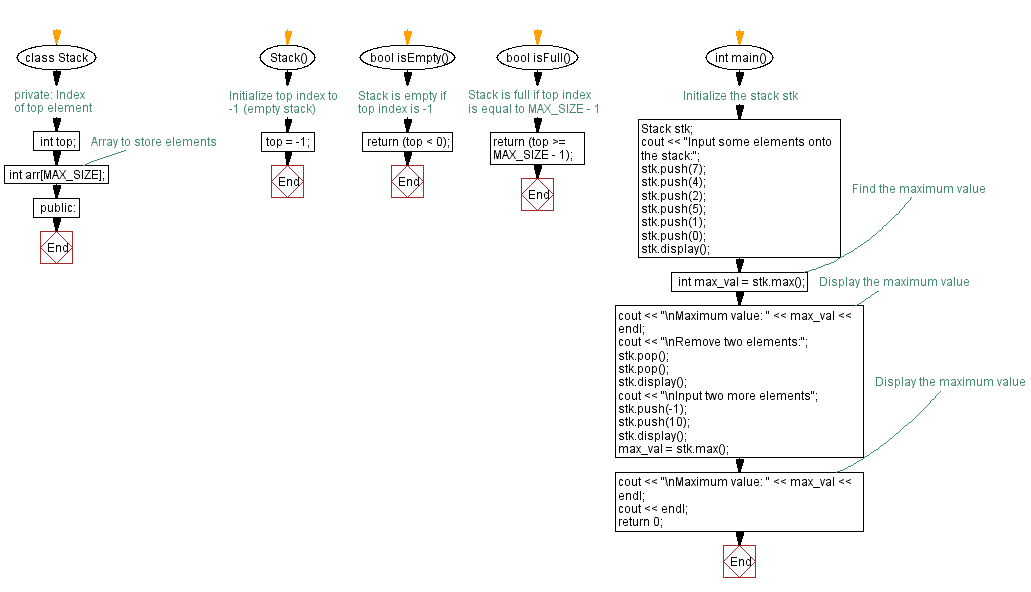
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Average value of the stack elements (using an array).
Next C++ Exercise: Find the minimum element in a stack (using an array).
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/stack/cpp-stack-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics