C++ String Exercises: Find the numbers in a string and calculate the sum
14. Sum of Numbers in a String
Write a C++ program to find the numbers in a given string and calculate the sum of all numbers.
Visual Presentation:
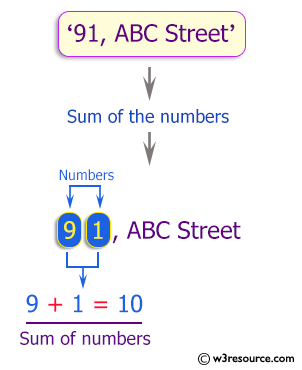
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string library for string operations
using namespace std; // Using the standard namespace
// Function to find numbers in a string and calculate their sum
int find_nums_and_sum(string str) {
int sum_num = 0; // Initializing the variable to store the sum of numbers found
string temp_str; // Creating a temporary string to store consecutive digits
// Loop through each character in the string
for (int x = 0; x < str.length(); x++)
{
// Check if the character is a digit
if (isdigit(str[x]))
{
temp_str.push_back(str[x]); // Add the digit to the temporary string
// Loop to find consecutive digits in the string
for (int y = x + 1; y < str.length(); y++)
{
// Check if the index exceeds the string length
if (y >= str.length())
{
break;
}
// Check if the next character is a digit
else if (isdigit(str[y]))
{
temp_str.push_back(str[y]); // Add the digit to the temporary string
x = y; // Update the current index to the next digit
}
else
{
break;
}
}
sum_num += stoi(temp_str); // Convert the temporary string to an integer and add to the sum
temp_str.clear(); // Clear the temporary string for the next sequence of digits
}
}
return sum_num; // Return the sum of numbers found in the string
}
// Main function
int main() {
// Output the original strings and their corresponding sum of numbers found
cout << "Original string: 91, ABC Street.-> Sum of the numbers: "<< find_nums_and_sum("91, ABC Street.") << endl;
cout << "Original string: w3resource from 2008-> Sum of the numbers: "<< find_nums_and_sum("w3resource from 2008") << endl;
cout << "Original string: C++ Versiuon 14aa11bb23-> Sum of the numbers: "<< find_nums_and_sum("C++ Versiuon 14aa11bb23") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Original string: 91, ABC Street.-> Sum of the numbers: 91 Original string: w3resource from 2008-> Sum of the numbers: 2011 Original string: C++ Versiuon 14aa11bb23-> Sum of the numbers: 48
Flowchart:
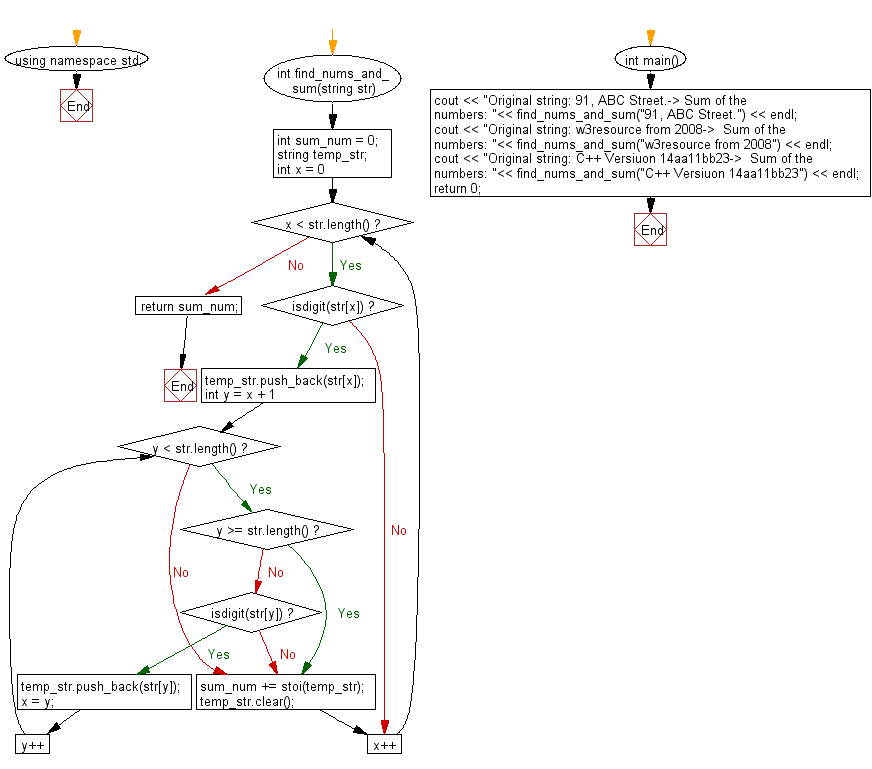
For more Practice: Solve these Related Problems:
- Write a C++ program to extract all numeric substrings from a given string and calculate their total sum.
- Write a C++ program that reads a string containing numbers and letters, then finds and sums all the number sequences.
- Write a C++ program to scan a string for digits, group them into numbers, and compute the sum of all found numbers.
- Write a C++ program that parses an alphanumeric string, extracts numbers using isdigit checks, and outputs the cumulative sum.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Change the case of each character of a given string.
Next C++ Exercise: Convert a given non-negative integer to English words.
What is the difficulty level of this exercise?