C++ String Exercises: Remove a specific character from a given string
38. Remove a Specific Character from a String
Write a C++ program that removes a specific character from a given string. Return the updated string.
Test Data:
("Filename", "e") -> "Filnam"
("Compilation Time", "i") -> "Complaton Tme"
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to remove all occurrences of a specific character from a string
string test(string str, char ch) {
std::string result_str; // Initialize an empty string to store the modified string
result_str.reserve(str.size()); // Reserve memory to optimize string appending
for (size_t i = 0; i < str.size(); ++i) { // Loop through each character in the input string
if (str[i] != ch) { // Check if the character is not equal to the specified character
result_str += str[i]; // Append the character to the result string if it's different from 'ch'
}
}
return result_str; // Return the modified string after removing occurrences of 'ch'
}
int main() {
// Test cases
string str = "Filename"; // Define a string
char ch = 'e'; // Define a character
cout << "String: " << str;
cout << "\nCharacter: " << ch;
cout << "\nRemove '" << ch << "' from '" << str << "': " << test(str, ch) << endl; // Display the string after removing 'e' from "Filename"
str = "Compilation Time"; // Update the string
ch = 'i'; // Update the character
cout << "\nString: " << str;
cout << "\nCharacter: " << ch;
cout << "\nRemove '" << ch << "' from '" << str << "': " << test(str, ch) << endl; // Display the string after removing 'i' from "Compilation Time"
}
Sample Output:
String: Filename Character: e Remove 'e' from 'Filename': Filnam String: Compilation Time Character: i Remove 'i' from 'Compilation Time': Complaton Tme
Flowchart:
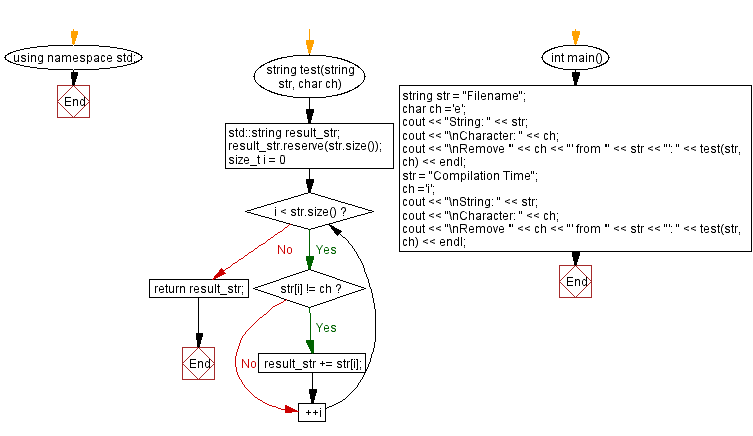
For more Practice: Solve these Related Problems:
- Write a C++ program to remove all instances of a given character from a string without using library functions.
- Write a C++ program that reads a string and a character, then outputs the string after eliminating that character.
- Write a C++ program to scan a string and build a new string that omits a specified character entirely.
- Write a C++ program to process an input string and filter out a target character using pointer arithmetic.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count occurrences of a certain character in a string.
Next C++ Exercise: Find Unique Character String.What is the difficulty level of this exercise?