C#: Sort elements of the array in descending order
Write a C# Sharp program to sort array elements in descending order.
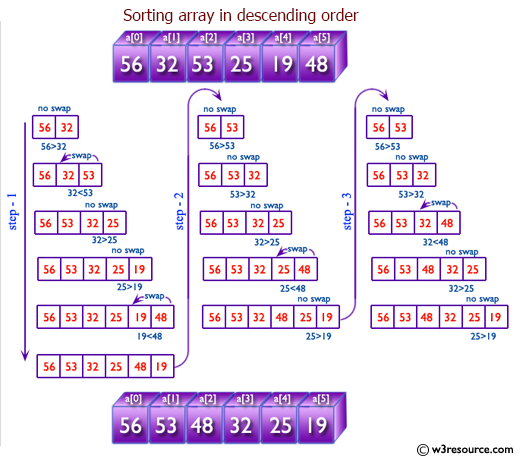
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise12
{
public static void Main()
{
int[] arr1 = new int[10]; // Declare an array to store integers
int n, i, j, tmp; // Declare variables for array size, counting, and temporary storage
// Display a message prompting the user to input the size of the array
Console.Write("\n\nsort elements of array in descending order :\n");
Console.Write("----------------------------------------------\n");
Console.Write("Input the size of array : ");
n = Convert.ToInt32(Console.ReadLine()); // Read the size of the array entered by the user
// Prompt the user to input 'n' elements in the array
Console.Write("Input {0} elements in the array :\n", n);
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Store user input in the array
}
// Sort the elements of the array in descending order using nested loops and swapping if needed
for (i = 0; i < n; i++)
{
for (j = i + 1; j < n; j++)
{
if (arr1[i] < arr1[j]) // Compare elements to arrange them in descending order
{
tmp = arr1[i]; // Swap elements if the condition is met
arr1[i] = arr1[j];
arr1[j] = tmp;
}
}
}
// Display the sorted elements of the array in descending order
Console.Write("\nElements of the array in sorted descending order:\n");
for (i = 0; i < n; i++)
{
Console.Write("{0} ", arr1[i]); // Print sorted elements
}
Console.Write("\n\n"); // Print a new line for formatting
}
}
Sample Output:
sort elements of array in descending order : ---------------------------------------------- Input the size of array : 3 Input 3 elements in the array : element - 0 : 2 element - 1 : 5 element - 2 : 0 Elements of the array in sorted descending order: 5 2 0
Flowchart:
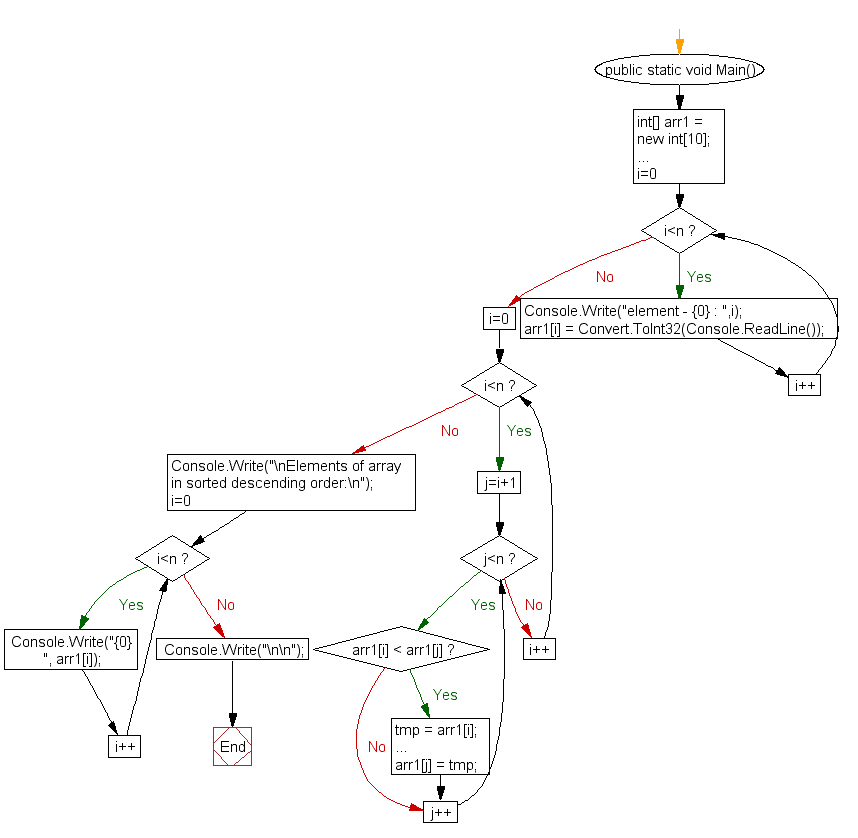
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to sort elements of array in ascending order.
Next: Write a program in C# Sharp to insert New value in the array (sorted list ).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.