C#: Find the product of two integers in an array
C# Sharp Array: Exercise-41 with Solution
Write a C# Sharp program to find two numbers in an array of integers whose product is equal to a given number.
Sample Data:
({10, 18, 39, 75, 100}, 180) -> {10, 18}
({10, 18, 39, 75, 100}, 200) -> {}
({10, 18, 39, 75, 100}, 702) -> {18, 39}
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums1 = { 10, 18, 39, 75, 100 };
// Displaying original array elements using string.Join method
Console.WriteLine("Original array elements:");
int n = 180;
Console.WriteLine($"{string.Join(", ", nums1)}");
// Displaying the given number 'n'
Console.WriteLine("The given number (n): " + n);
// Finding and displaying pairs of integers in the array that multiply to give 'n'
Console.WriteLine("Product of two integers in the said array equal to n:");
int[] result = test(nums1, n);
Console.WriteLine($"{string.Join(", ", result)}");
// Changing the value of 'n' and repeating the process
n = 200;
Console.WriteLine("\nOriginal array elements:");
Console.WriteLine($"{string.Join(", ", nums1)}");
Console.WriteLine("The given number (n): " + n);
Console.WriteLine("Product of two integers in the said array equal to n:");
int[] result1 = test(nums1, n);
Console.WriteLine($"{string.Join(", ", result1)}");
n = 702;
Console.WriteLine("\nOriginal array elements:");
Console.WriteLine($"{string.Join(", ", nums1)}");
Console.WriteLine("The given number (n): " + n);
Console.WriteLine("Product of two integers in the said array equal to n:");
int[] result2 = test(nums1, n);
Console.WriteLine($"{string.Join(", ", result2)}");
}
// Method to find pairs of integers in the array that multiply to give 'n'
public static int[] test(int[] nums, int n)
{
List<int> result = new List<int>();
// Loop through each element in the 'nums' array
foreach (int x in nums)
{
foreach (int y in nums)
{
// Check if the product of 'x' and 'y' equals 'n'
if (x * y == n)
{
// If the product equals 'n', add 'y' to the result list
result.Add(y);
}
}
}
// Sort the result list in ascending order and convert it to an array before returning
return result.OrderBy(el => el).ToArray();
}
}
}
Sample Output:
Original array elements: 10, 18, 39, 75, 100 The given number (n): 180 Product of two integers in the said array equal to n: 10, 18 Original array elements: 10, 18, 39, 75, 100 The given number (n): 200 Product of two integers in the said array equal to n: Original array elements: 10, 18, 39, 75, 100 The given number (n): 702 Product of two integers in the said array equal to n: 18, 39
Flowchart:
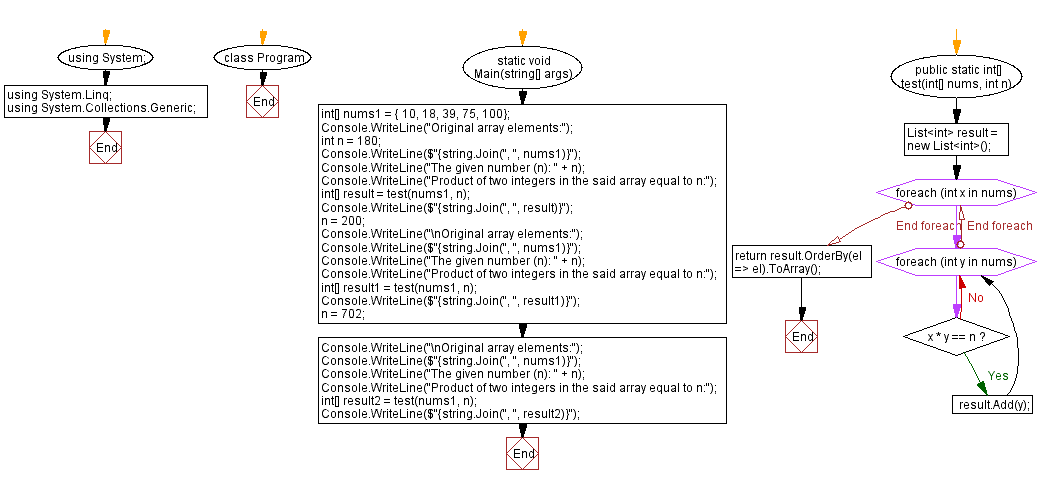
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous C# Sharp Exercise: Smallest positive which is not present in an array.
Next C# Sharp Exercise: C# Sharp Searching and Sorting Algorithm Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics