C#: Print all unique elements of an array
Write a program in C# Sharp to print all unique elements in an array.
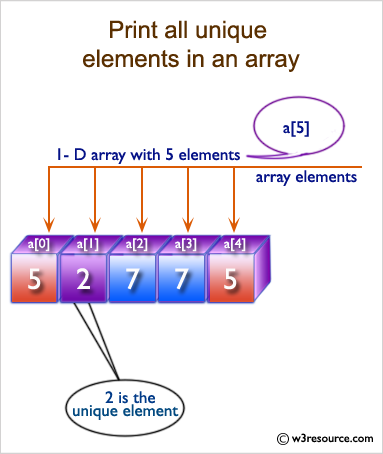
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise6
{
public static void Main()
{
int n, ctr = 0; // Declaration of variables 'n' (number of elements) and 'ctr' (counter)
int[] arr1 = new int[100]; // Declaration of an integer array 'arr1' with size 100
int i, j, k; // Declaration of loop control variables 'i', 'j', and 'k'
// Display a message to print all unique elements of an array
Console.Write("\n\nPrint all unique elements of an array:\n");
Console.Write("------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
n = Convert.ToInt32(Console.ReadLine()); // Read the number of elements from the user and store it in 'n'
Console.Write("Input {0} elements in the array :\n", n); // Prompt the user to input 'n' elements
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Read user input and store it in the array 'arr1'
}
// Checking for unique elements in the array
Console.Write("\nThe unique elements found in the array are : \n");
for (i = 0; i < n; i++)
{
ctr = 0; // Reset the counter for each element in the array
// Check for duplicates before the current position in the array
for (j = 0; j < i - 1; j++)
{
// Increment the counter if a duplicate is found
if (arr1[i] == arr1[j])
{
ctr++;
}
}
// Check for duplicates after the current position in the array
for (k = i + 1; k < n; k++)
{
// Increment the counter if a duplicate is found
if (arr1[i] == arr1[k])
{
ctr++;
}
// Skip if duplicate numbers are next to each other
if (arr1[i] == arr1[i + 1])
{
i++; // Increment 'i' to skip the next element
}
}
// Print the value if it's unique (counter remains 0)
if (ctr == 0)
{
Console.Write("{0} ", arr1[i]);
}
}
Console.Write("\n\n"); // Extra newline for formatting
}
}
Sample Output:
Print all unique elements of an array: ------------------------------------------ Input the number of elements to be stored in the array :2 Input 2 elements in the array : element - 0 : 2 element - 1 : 4 The unique elements found in the array are : 2 4
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count a total number of duplicate elements in an array.
Next: Write a program in C# Sharp to merge two arrays of same size sorted in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.