C#: Find maximum and minimum element in an array
Write a C# Sharp program to find the maximum and minimum elements in an array.
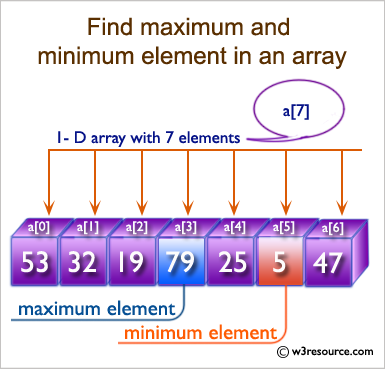
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise9
{
public static void Main()
{
int[] arr1 = new int[100]; // Array to store elements
int i, mx, mn, n; // Variables for maximum, minimum, and size of array
Console.Write("\n\nFind maximum and minimum element in an array :\n");
Console.Write("--------------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
n = Convert.ToInt32(Console.ReadLine()); // Read the number of elements
Console.Write("Input {0} elements in the array :\n", n);
for (i = 0; i < n; i++)
{
Console.Write("element - {0} : ", i);
arr1[i] = Convert.ToInt32(Console.ReadLine()); // Read elements into the array
}
// Initializing maximum and minimum with the first element of the array
mx = arr1[0];
mn = arr1[0];
// Finding maximum and minimum elements
for (i = 1; i < n; i++)
{
if (arr1[i] > mx) // If current element is greater than the current maximum
{
mx = arr1[i]; // Update maximum
}
if (arr1[i] < mn) // If current element is smaller than the current minimum
{
mn = arr1[i]; // Update minimum
}
}
// Display maximum and minimum elements
Console.Write("Maximum element is : {0}\n", mx);
Console.Write("Minimum element is : {0}\n\n", mn);
}
}
Sample Output:
Find maximum and minimum element in an array : -------------------------------------------------- Input the number of elements to be stored in the array :2 Input 2 elements in the array : element - 0 : 20 element - 1 : 25 Maximum element is : 25 Minimum element is : 20
Flowchart:
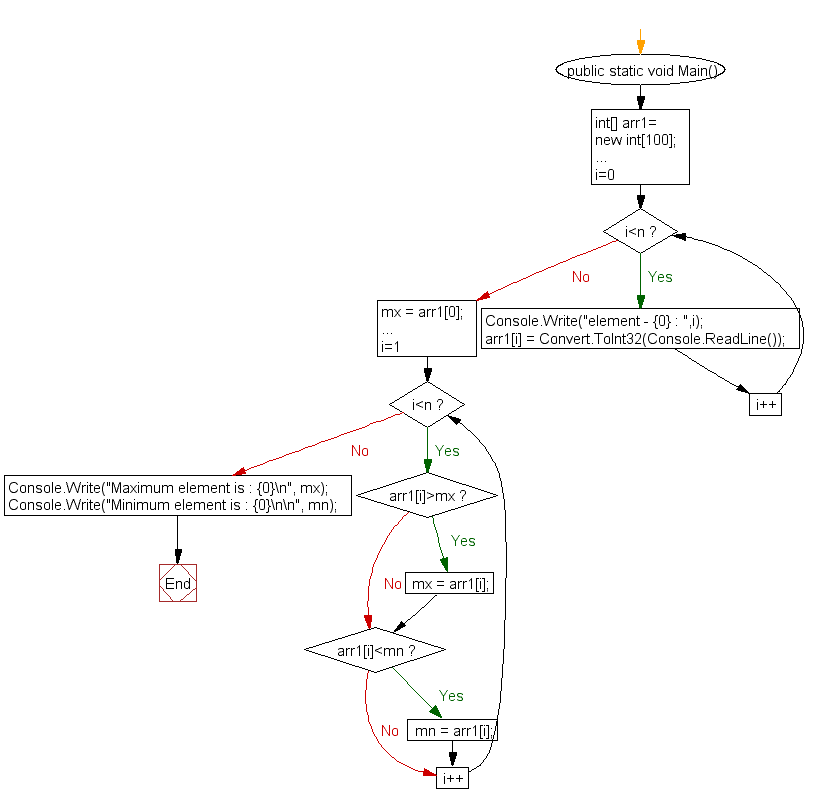
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count the frequency of each element of an array.
Next: Write a program in C# Sharp to separate odd and even integers in separate arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.