C#: Create an array taking two middle elements from a given array of integers of length even
Middle Elements of Even-Length Array
Write a C# Sharp program to create an array taking two middle elements from a given array of integers of length even.
Visual Presentation:
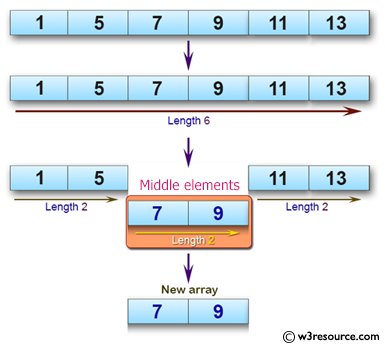
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 1, 5, 7, 9, 11, 13 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to create a new array containing the middle two elements of the input array
static int[] test(int[] numbers)
{
// Calculating the middle index and selecting the middle two elements
return new int[] { numbers[numbers.Length / 2 - 1], numbers[numbers.Length / 2] };
}
}
}
Sample Output:
New array: 7 9
Flowchart:
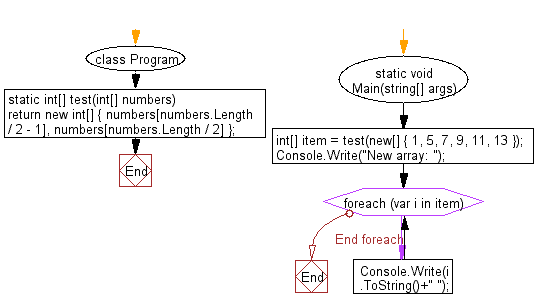
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
Next: Write a C# Sharp program to create a new array from two give array of integers , each length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.