C#: Shift an element in left direction and return a new array
Shift Elements Left in Array
Write a C# Sharp program to shift an element left and return an array.
Visual Presentation:
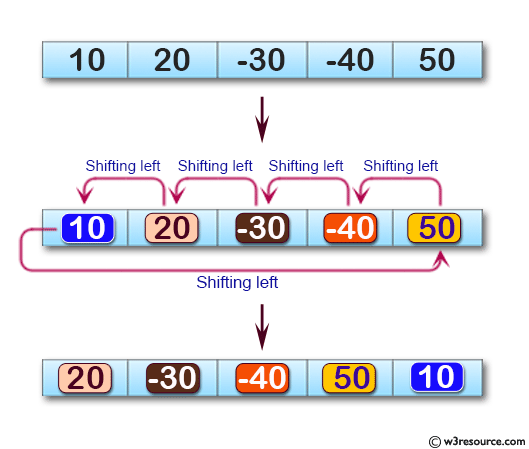
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array and storing the returned array in 'item'
int[] item = test(new[] { 10, 20, -30, -40, 50 });
// Outputting the elements of the new array after shifting
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString() + " "); // Outputting each element followed by a space
}
}
// Method to shift elements in an array to the left
static int[] test(int[] numbers)
{
int size = numbers.Length; // Storing the length of the input array
// Creating a new array to hold the shifted elements
int[] shiftNums = new int[size];
// Looping through each element of the input array
for (int i = 0; i < size; i++)
{
// Shifting the elements to the left using modulo operation to handle circular shifting
shiftNums[i] = numbers[(i + 1) % size];
}
return shiftNums; // Returning the new array with shifted elements
}
}
}
Sample Output:
New array: 20 -30 -40 50 10
Flowchart:
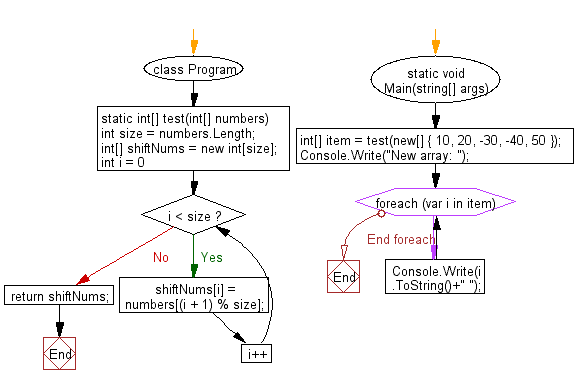
Go to:
PREV : Check for Three Increasing Adjacent Numbers.
NEXT : Elements Before 5 in Array.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.