C#: Find the larger average value between the first and the second half of a given array of integers
Largest Average Between Array Halves
Write a C# Sharp program to find the largest average value between the first and second half of a given array of integers. Minimum length is at least 2. Assume that the second half begins at index (array length)/2.
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
using System.Collections; // Importing collections for non-generic interfaces
using System.Collections.Generic; // Importing collections for generic interfaces
using System.Linq; // Importing LINQ for querying collections
using System.Text; // Importing classes for handling strings
using System.Threading.Tasks; // Importing classes for handling asynchronous tasks
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays
Console.WriteLine(test(new[] { 1, 2, 3, 4, 6, 8 }));
Console.WriteLine(test(new[] { 15, 2, 3, 4, 15, 11 }));
}
// Method to calculate the average of the first and second halves of an array and return the greater average
static int test(int[] numbers)
{
// Calculating the average of the first and second halves of the array
var firstHalf = Average(numbers, 0, numbers.Length / 2);
var secondHalf = Average(numbers, numbers.Length / 2, numbers.Length);
// Returning the greater of the two averages
return firstHalf > secondHalf ? firstHalf : secondHalf;
}
// Method to calculate the average of a specified range in the array
private static int Average(int[] num, int start, int end)
{
var sum = 0; // Variable to store the sum of elements within the specified range
// Loop to sum elements within the specified range
for (var i = start; i < end; i++)
sum += num[i];
// Calculating and returning the average of the elements in the specified range
return sum / (num.Length / 2); // Here, it's dividing by (num.Length / 2), but it should be (end - start)
}
}
}
Sample Output:
6 10
Flowchart: 1
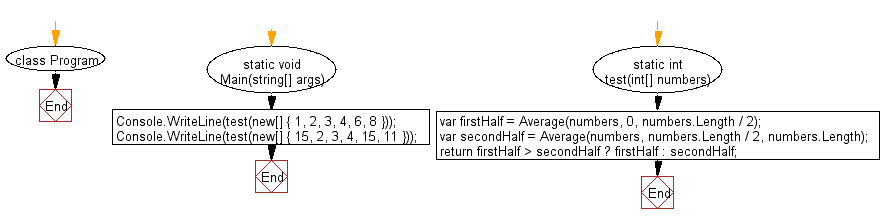
Flowchart: 2
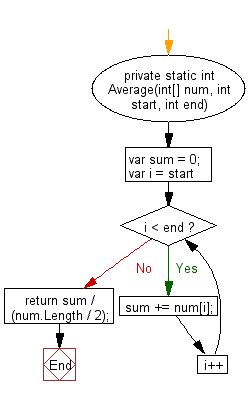
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array (length will be atleast 2) of integers and return true if there are two values 15, 15 next to each other.
Next: Write a C# Sharp program to count the number of strings with given length in given array of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.