C#: Create a new list from a given list of strings where each element has "#" added at the beginning and end position
C# Sharp Basic Algorithm: Exercise-143 with Solution
Add # at Start and End of Strings
Write a C# Sharp program to create a list from a given list of strings. Each element has "#" added at the beginning and end position.
Visual Presentation:
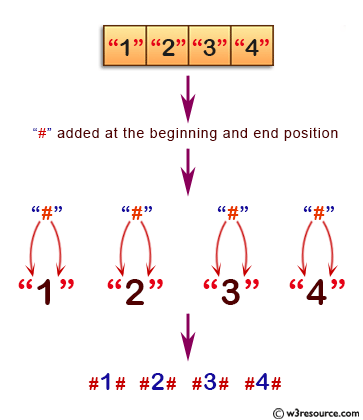
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Creating a List of strings and assigning the result of the 'test' method
List<string> mylist = test(new List<string>(new string[] { "1", "2", "3", "4" }));
// Displaying the elements of 'mylist' in the console
foreach (var i in mylist)
{
Console.Write(i.ToString() + " "); // Output each element followed by a space
}
}
// Method that takes a List of strings and adds '#' at the beginning and end of each string
public static List<string> test(List<string> nums_str)
{
// Using LINQ to append '#' at the start and end of each string in 'nums_str'
IEnumerable<string> s = nums_str.Select(x => "#" + x + "#");
// Converting the IEnumerable 's' to a new List and returning it
return s.ToList();
}
}
}
Sample Output:
#1# #2# #3# #4#
Flowchart:
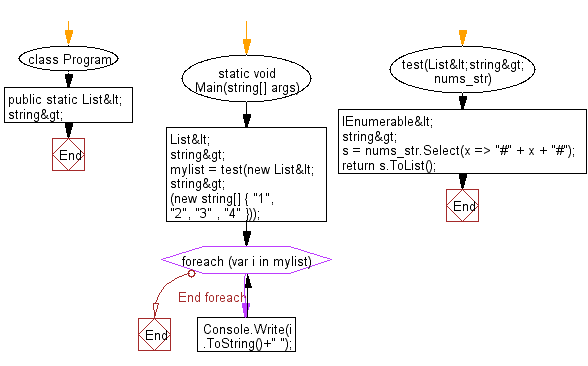
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of integers where each integer multiplied by itself three times.
Next: Write a C# Sharp program to create a new list from a given list of strings where each element is replaced by 4 copies of the string concatenated together.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-143.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics