C#: Check which number is nearest to the value 100 among two given integers
Closest to 100 Between Two
Write a C# Sharp program to check which number is closest to 100 among two given integers. Return 0 if the two numbers are equal.
Visual Presentation 1:
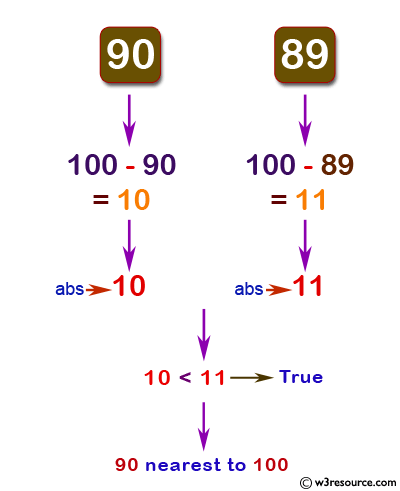
Visual Presentation 2:
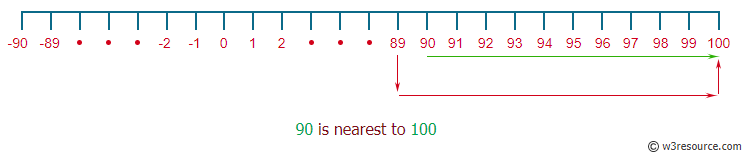
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different integer values
Console.WriteLine(test(78, 95)); // Output: 95
Console.WriteLine(test(95, 95)); // Output: 0
Console.WriteLine(test(99, 70)); // Output: 99
Console.ReadLine(); // Keeping the console window open
}
// Method to compare two integers and return the closest to 100
public static int test(int x, int y)
{
// Constant integer 'n' is assigned the value 100
const int n = 100;
// Calculate the absolute difference between 'x' and 'n'
var val = Math.Abs(x - n);
// Calculate the absolute difference between 'y' and 'n'
var val2 = Math.Abs(y - n);
// Check if the differences are equal; if true, return 0 as both 'x' and 'y' are equidistant from 100
// Otherwise, return the number that is closer to 100 (the one with the smaller absolute difference)
return val == val2 ? 0 : (val < val2 ? x : y);
}
}
}
Sample Output:
95 0 99
Flowchart:
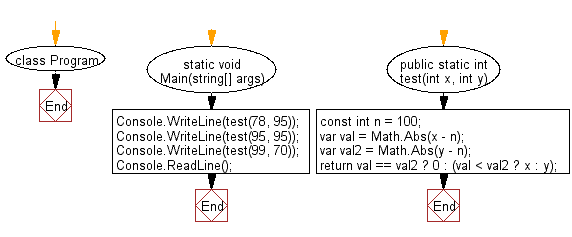
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check the largest number among three given integers.
Next: Write a C# Sharp program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.