C#: Find the larger value from two positive integer values that is in the range 20..30 inclusive
C# Sharp Basic Algorithm: Exercise-21 with Solution
Largest in Range 20-30
Write a C# Sharp program to find the largest value from two positive integer values in the range 20..30 inclusive. Return 0 if neither is in that range.
Visual Presentation:
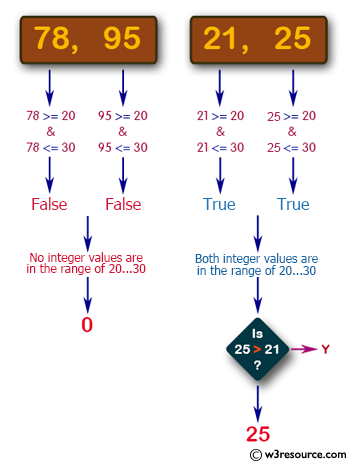
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different integer values
Console.WriteLine(test(78, 95)); // Output: 95
Console.WriteLine(test(20, 30)); // Output: 30
Console.WriteLine(test(21, 25)); // Output: 25
Console.WriteLine(test(28, 28)); // Output: 28
Console.ReadLine(); // Keeping the console window open
}
// Method to compare two integers and return the larger one based on certain conditions
public static int test(int x, int y)
{
// Check if both x and y are within the range [20, 30]
if (x >= 20 && x <= 30 && y >= 20 && y <= 30)
{
// If x is greater than or equal to y, return x
if (x >= y)
{
return x;
}
else // If y is greater than x, return y
{
return y;
}
}
else if (x >= 20 && y <= 30) // If x is within the range [20, 30], return x
{
return x;
}
else if (y >= 20 && y <= 30) // If y is within the range [20, 30], return y
{
return y;
}
else // If neither x nor y is within the range [20, 30], return 0
{
return 0;
}
}
}
}
Sample Output:
0 30 25 28
Flowchart:
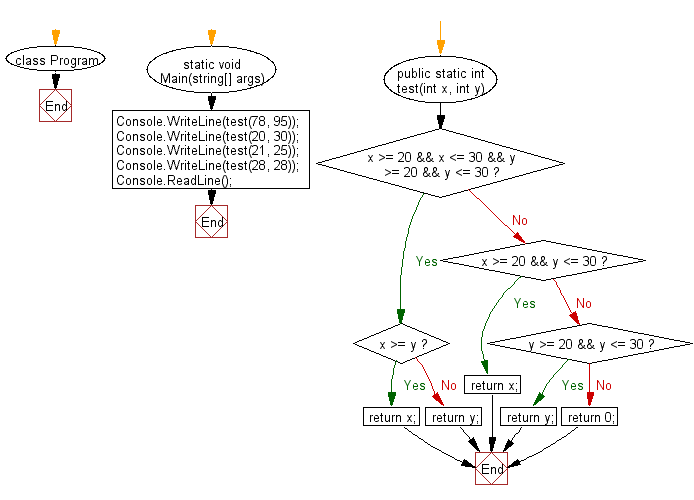
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
Next: Write a C# Sharp program to check if a given string contains between 2 and 4 'z' character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-21.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics