C#: Convert the last 3 characters of a given string in upper case
Uppercase Last Three Characters
Write a C# Sharp program to convert the last 3 characters of a given string to uppercase. If the length of the string is less than 3, then uppercase all the characters.
Visual Presentation:
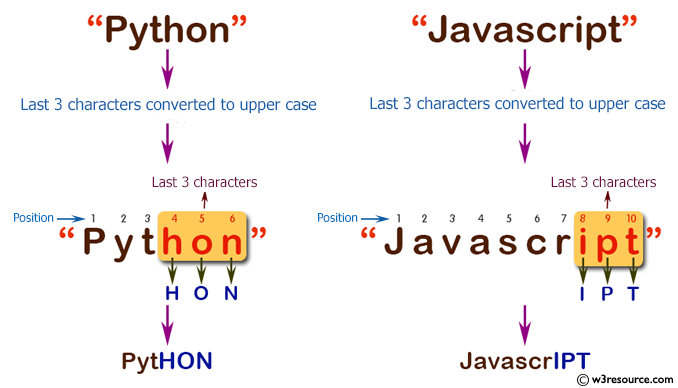
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Printing results of the test method with various inputs
Console.WriteLine(test("Python"));
Console.WriteLine(test("Javascript"));
Console.WriteLine(test("js"));
Console.WriteLine(test("PHP"));
Console.ReadLine(); // Allowing user to read the output before the console closes
}
// Method to modify a string according to the condition
public static string test(string str)
{
// Ternary operator: If the length of the string is less than 3, return the uppercase string.
// Otherwise, remove the last 3 characters, convert them to uppercase, and append them back.
return str.Length < 3 ? str.ToUpper() : str.Remove(str.Length - 3) + str.Substring(str.Length - 3).ToUpper();
}
}
}
Sample Output:
PytHON JavascrIPT JS PHP
Flowchart:
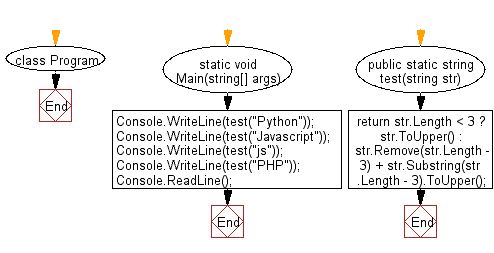
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if two given non-negative integers have the same last digit.
Next: Write a C# Sharp program to create a new string which is n (non-negative integer ) copies of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.