C#: Create a new string from a given string where a specified character have been removed except starting and ending position of the given string
C# Sharp Basic Algorithm: Exercise-36 with Solution
Remove Character Except First and Last
Write a C# Sharp program to create a string from a given string where a specified character is removed except for the beginning and ending positions.
Visual Presentation:
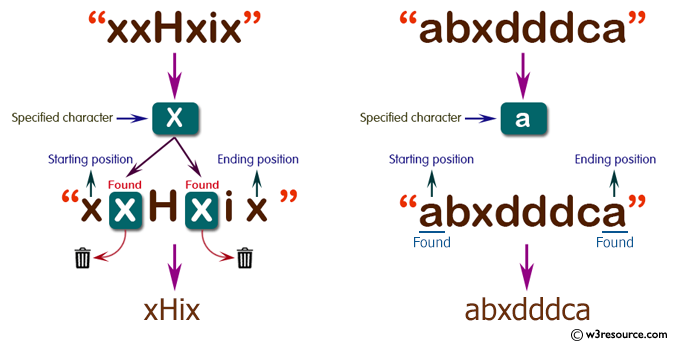
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'StringX' method with different strings and characters
Console.WriteLine(StringX("xxHxix", "x")); // Output: xHix
Console.WriteLine(StringX("abxdddca", "a")); // Output: bxdddc
Console.WriteLine(StringX("xabjbhtrb", "b"));// Output: xajhtr
Console.ReadLine(); // Keeping the console window open
}
// Method to remove occurrences of a specific character 'c' except for the first and last occurrences in a string 'str1'
public static string StringX(string str1, string c)
{
// Iterate through the characters in the string 'str1' starting from the second last character
for (int i = str1.Length - 2; i > 0; i--)
{
// Check if the current character is equal to the given character 'c'
if (str1[i] == c[0])
{
// If so, remove that character from the string 'str1'
str1 = str1.Remove(i, 1);
}
}
return str1; // Return the modified string 'str1'
}
}
}
Sample Output:
xHix abxdddca xajhtrb
Flowchart:
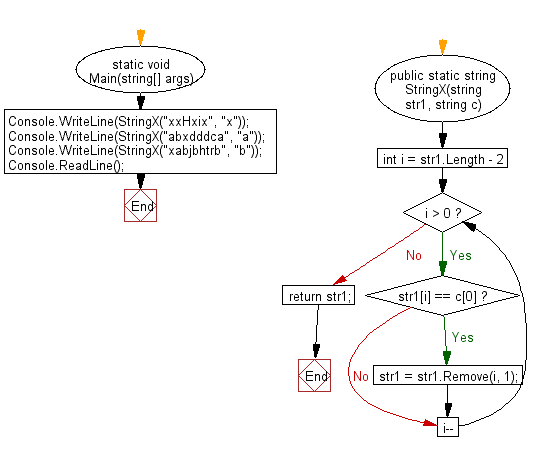
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to comapre two given strings and return the number of the positions where they contain the same length 2 substring.
Next: Write a C# Sharp program to create a new string of the characters at indexes 0,1, 4,5, 8,9 ... from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-36.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics