C#: Check a given integer and return true if it is within 10 of 100 or 200
Within 10 of 100 or 200
Write a C# Sharp program to check a given integer and return true if it is within 10 of 100 or 200.
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method and displaying the returned boolean values
Console.WriteLine(test(103)); // Output: True
Console.WriteLine(test(90)); // Output: True
Console.WriteLine(test(89)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if a given integer is within 10 units of 100 or 200
public static bool test(int x)
{
// Checking if the absolute difference between 'x' and 100 is less than or equal to 10,
// OR the absolute difference between 'x' and 200 is less than or equal to 10.
// If either condition is true, returns true; otherwise, returns false.
if (Math.Abs(x - 100) <= 10 || Math.Abs(x - 200) <= 10)
return true;
return false;
}
}
}
Sample Output:
True True False
Flowchart:
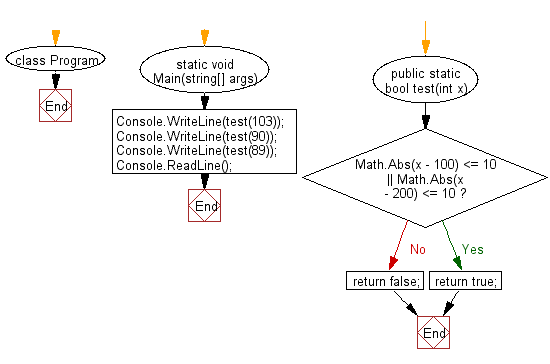
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Next: Write a C# Sharp program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.