C#: Check whether three given numbers are in strict increasing order
Strict Increasing Order for Three Numbers
Write a C# Sharp program to check if three given numbers are in strict increasing order.
For example, 4 7 15, or 45, 56, 67, but not 4 ,5, 8 or 6, 6, 8. However, if a fourth parameter is true, equality is allowed, such as 6, 6, 8 or 7, 7, 7.
Visual Presentation:
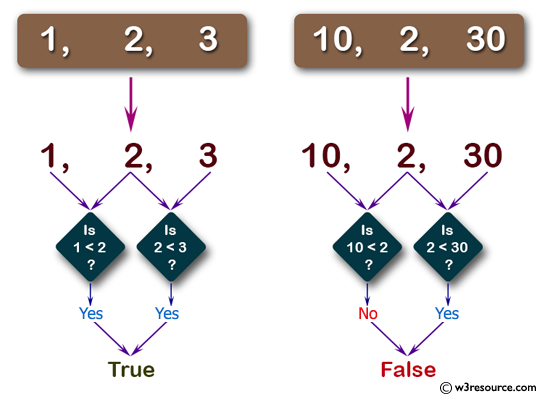
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integer values and a boolean flag, and printing the results
Console.WriteLine(test(1, 2, 3, false)); // Output: True (since flag is false, checks strict ascending order)
Console.WriteLine(test(1, 2, 3, true)); // Output: True (since flag is true, checks non-strict ascending order)
Console.WriteLine(test(10, 2, 30, false)); // Output: False (since flag is false, checks strict ascending order)
Console.WriteLine(test(10, 10, 30, true)); // Output: True (since flag is true, checks non-strict ascending order)
Console.ReadLine(); // Keeping the console window open
}
// Method to check the order of integers based on the flag value
public static bool test(int x, int y, int z, bool flag)
{
// If flag is true, checks for non-strict ascending order (x <= y <= z)
// If flag is false, checks for strict ascending order (x < y < z)
return flag ? x <= y && y <= z : x < y && y < z;
}
}
}
Sample Output:
True True False True
Flowchart:
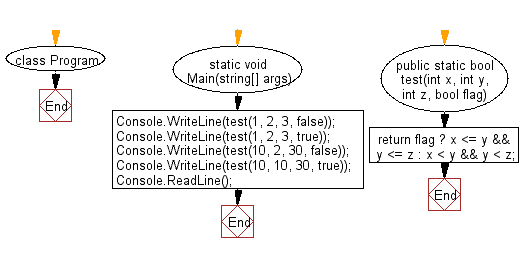
For more Practice: Solve these Related Problems:
- Write a C# program to check if numbers are non-decreasing, allowing duplicates only if the flag is true.
- Write a C# program to return true if the numbers are in non-increasing order or equal when a flag is set.
- Write a C# program to check whether a set of four numbers is strictly increasing, unless a flag permits equal values.
- Write a C# program to validate increasing order across integers with a flag to allow reverse order.
Go to:
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
PREV : Strict Increasing Order for Three Numbers.
NEXT : Write a C# Sharp program to check if two or more non-negative given integers have the same rightmost digit
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.