C#: Check three given integers and return true if the difference between small and medium and the difference between medium and large is same
C# Sharp Basic Algorithm: Exercise-59 with Solution
Check Equal Differences in Three Integers
Write a C# Sharp program to compare three integers (small, medium, and large) and return true if the difference between small and medium and the difference between medium and large is the same.
Visual Presentation:
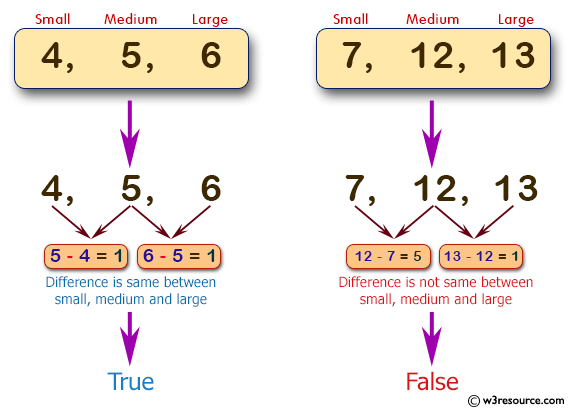
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
using System.Collections.Generic; // Import the System.Collections.Generic namespace for collections
using System.Linq; // Import the LINQ namespace for LINQ functionality
using System.Text; // Import the System.Text namespace for string manipulation
using System.Threading.Tasks; // Import the System.Threading.Tasks namespace for asynchronous operations
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of test function with three integers as arguments
Console.WriteLine(test(4, 5, 6)); // Test with integers 4, 5, and 6
Console.WriteLine(test(7, 12, 13)); // Test with integers 7, 12, and 13
Console.WriteLine(test(-1, 0, 1)); // Test with integers -1, 0, and 1
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes three integers 'x', 'y', and 'z' and returns a boolean value
public static bool test(int x, int y, int z)
{
// Conditions to check the arithmetic sequence property between the three integers
if (x > y && x > z && y > z) return x - y == y - z; // Check if x > y > z
if (x > y && x > z && z > y) return x - z == z - y; // Check if x > z > y
if (y > x && y > z && x > z) return y - x == x - z; // Check if y > x > z
if (y > x && y > z && z > x) return y - z == z - x; // Check if y > z > x
if (z > x && z > y && x > y) return z - x == x - y; // Check if z > x > y
return z - y == y - x; // If none of the above conditions match, check if z > y > x
}
}
}
Sample Output:
True False True
Flowchart:
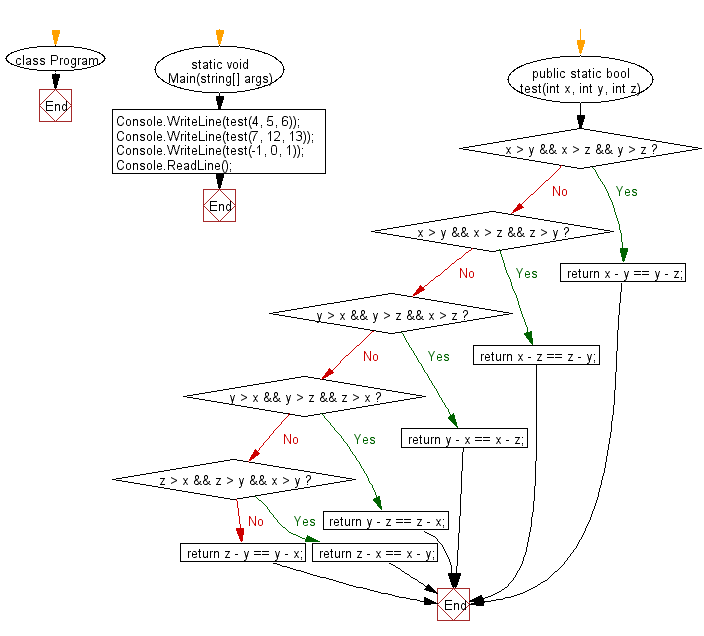
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Next: Write a C# Sharp program to create a new string using two given strings s1, s2, the format of the new string will be s1s2s2s1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-59.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics