C#: Create a new string using first two characters of a given string
First Two Characters or Original String
Write a C# Sharp program to create a new string using the first two characters of a given string. If the string length is less than 2, return the original string.
Visual Presentation:
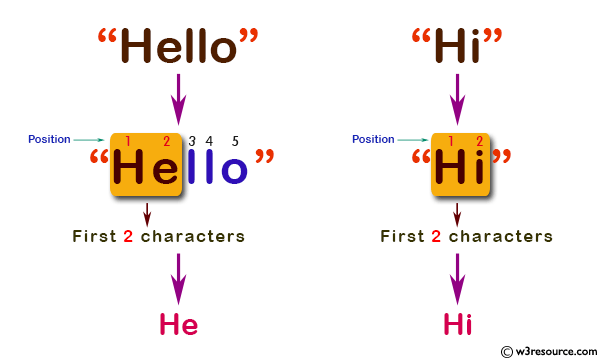
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Test with the string "Hello"
Console.WriteLine(test("Hi")); // Test with the string "Hi"
Console.WriteLine(test("H")); // Test with the string "H"
Console.WriteLine(test(" ")); // Test with a single space string
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes a string 's1' and returns a string
public static string test(string s1)
{
// Check if the length of the input string 's1' is less than 2
// If true, return the input string 's1' as it is
// If false, return the substring of 's1' starting from index 0 up to index 1 (two characters)
return s1.Length < 2 ? s1 : s1.Substring(0, 2);
}
}
}
Sample Output:
He Hi H
Flowchart:
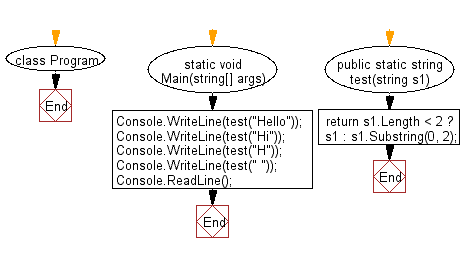
Go to:
PREV : Three Copies of Last Two Characters.
NEXT : First Half of Even-Length String.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.