C#: Create a new string from two given string one is shorter and another is longer
Format Long+Short+Long Strings
Write a C# Sharp program to create a new string from two given strings, one of which is shorter and one of which is longer. The new string format will be long string + short string + long string.
Visual Presentation:
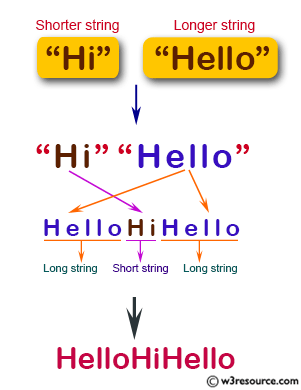
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the test function with different strings as arguments
Console.WriteLine(test("Hello", "Hi")); // Test with strings "Hello" and "Hi"
Console.WriteLine(test("JS", "Python")); // Test with strings "JS" and "Python"
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes two strings 's1' and 's2' and returns a string
public static string test(string s1, string s2)
{
// Check if the length of 's1' is less than the length of 's2'
// If true, concatenate 's2', 's1', and 's2'
// If false, concatenate 's1', 's2', and 's1'
return s1.Length < s2.Length ? s2 + s1 + s2 : s1 + s2 + s1;
}
}
}
Sample Output:
HelloHiHello PythonJSPython
Flowchart:
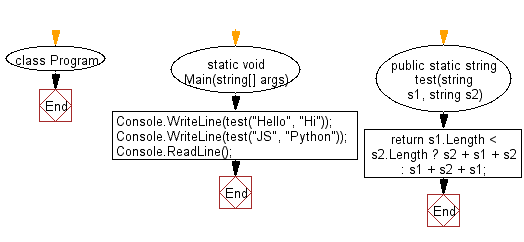
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string without the first and last character of a given string of length atleast two.
Next: Write a C# Sharp program to combine two given string of length atleast 1, after removing their first character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.