C#: Move the first two characters to the end of a given string of length at least two
Move First Two Characters to End
Write a C# Sharp program to move the first two characters to the end of a given string of length at least two.
Visual Presentation:
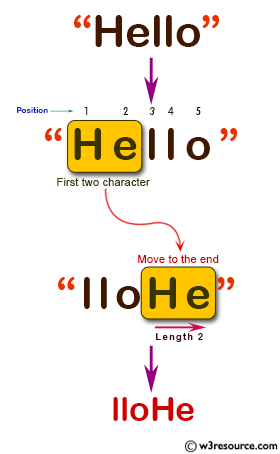
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Test with the string "Hello"
Console.WriteLine(test("JS")); // Test with the string "JS"
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes a string 's1' and returns a string
public static string test(string s1)
{
// Remove the first 2 characters from 's1' using Remove(startIndex, count)
// Then concatenate the removed characters at the end using Substring(startIndex, length)
return s1.Remove(0, 2) + s1.Substring(0, 2);
}
}
}
Sample Output:
lloHe JS
Flowchart:
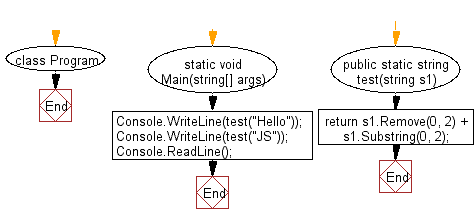
Go to:
PREV : Combine Strings After Removing First Char.
NEXT : Move Last Two Characters to Start.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.