C#: Check whether a given string ends with "on"
C# Sharp Basic Algorithm: Exercise-72 with Solution
Ends with 'on'
Write a C# Sharp program to check whether a given string ends with "on".
Visual Presentation:
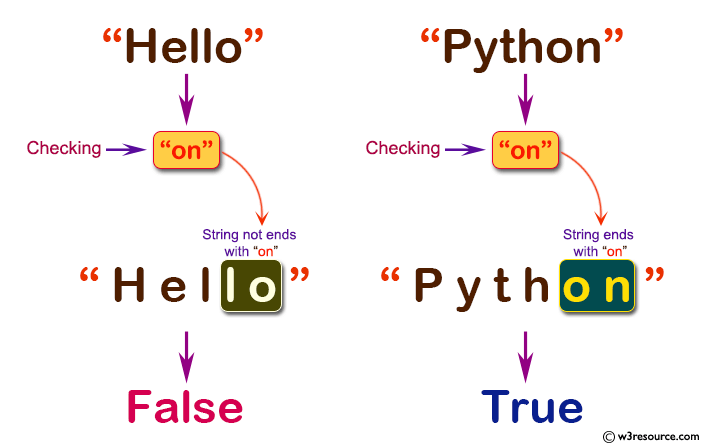
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Check if "Hello" ends with "on"
Console.WriteLine(test("Python")); // Check if "Python" ends with "on"
Console.WriteLine(test("on")); // Check if "on" ends with "on"
Console.WriteLine(test("o")); // Check if "o" ends with "on"
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes a string 's1' and returns a boolean value
public static bool test(string s1)
{
// Check if the string 's1' ends with the substring "on"
return s1.EndsWith("on");
}
}
}
Sample Output:
False True True False
Flowchart:
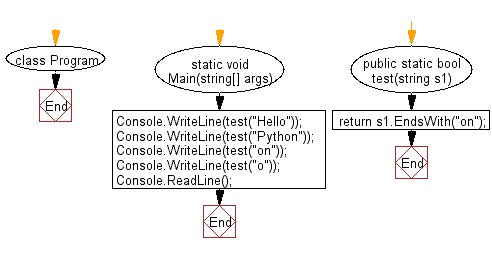
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string using the two middle characters of a given string of even length (at least 2).
Next: Write a C# Sharp program to create a new string using the first and last n characters from a given string of length at least n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-72.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics