C#: Create a new string taking 3 characters from the middle of a given string at least 3
Three Characters from Middle of String
Write a C# Sharp program to create a string by taking at least 3 characters from the middle of a given string.
Visual Presentation:
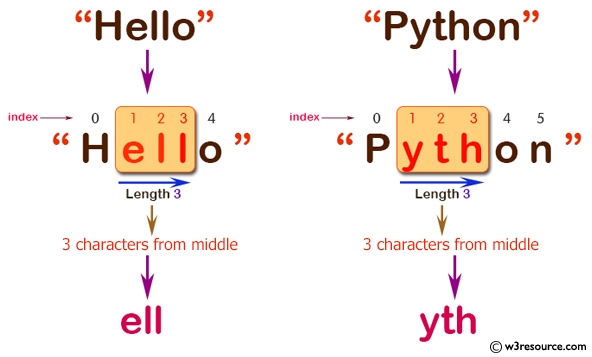
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Pass "Hello" as an argument to the test function
Console.WriteLine(test("Python")); // Pass "Python" as an argument to the test function
Console.WriteLine(test("abc")); // Pass "abc" as an argument to the test function
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes a string 's1' and returns a string
public static string test(string s1)
{
// Calculate the starting index to get a substring of length 3 from the middle of the string 's1'
// The formula (s1.Length - 1) / 2 - 1 calculates the starting index of the desired substring
return s1.Substring((s1.Length - 1) / 2 - 1, 3);
}
}
}
Sample Output:
ell yth abc
Flowchart:
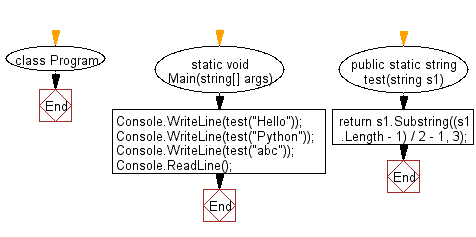
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string of length 2 starting at the given index of a given string.
Next: Write a C# Sharp program to create a new string of length 2, using first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.