C#: Create a new string using 3 copies of the first 2 characters of a given string
Three Copies of First Two Characters
Write a C# Sharp program to create a new string using 3 copies of the first 2 characters of a given string. If the string length is less than 2 use the whole string.
Visual Presentation:
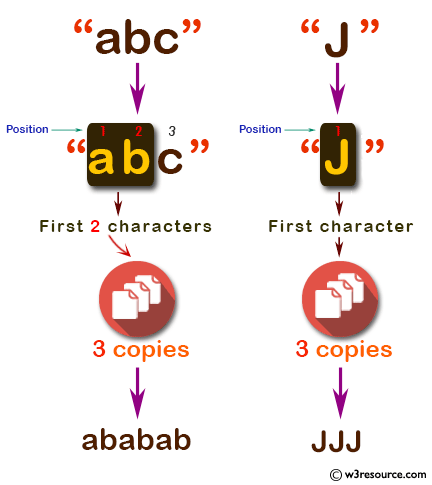
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("abc")); // Pass "abc" to the test function and display the result
Console.WriteLine(test("Python")); // Pass "Python" to the test function and display the result
Console.WriteLine(test("J")); // Pass "J" to the test function and display the result
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes a string 's1' as input and returns a string
public static string test(string s1)
{
string extra_Front = String.Empty; // Declaring an empty string variable 'extra_Front'
if (s1.Length < 2) // If the length of 's1' is less than 2
{
return s1 + s1 + s1; // Return 's1' concatenated with itself three times
}
else // If the length of 's1' is 2 or more
{
extra_Front = s1.Substring(0, 2); // Extract the first two characters of 's1' and assign them to 'extra_Front'
return extra_Front + extra_Front + extra_Front; // Return 'extra_Front' concatenated with itself three times
}
}
}
}
Sample Output:
ababab PyPyPy JJJ
Flowchart:
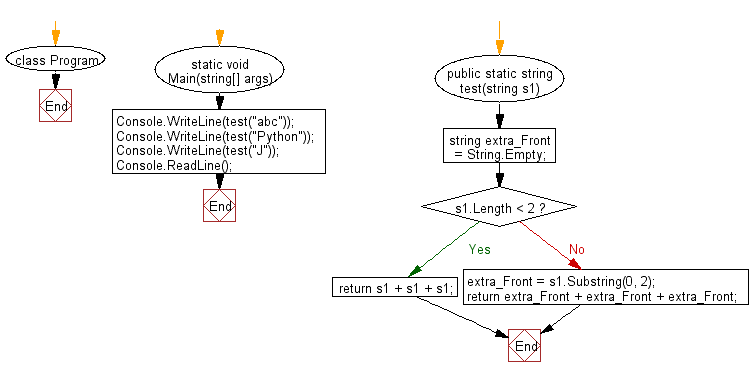
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to concate two given strings. If the given strings have different length remove the characters from the longer string.
Next: Write a C# Sharp program to create a new string from a given string. If the two characters of the given string from its beginning and end are same return the given string without the first two characters otherwise return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.