C#: Check a given array of integers of length 1 or more and return true if 10 appears as either first or last element in the given array
C# Sharp Basic Algorithm: Exercise-88 with Solution
10 as First or Last Element in Array
Write a C# Sharp program to check a given array of integers of length 1 or more. Return true if 10 appears as either the first or last element in the given array.
Visual Presentation:
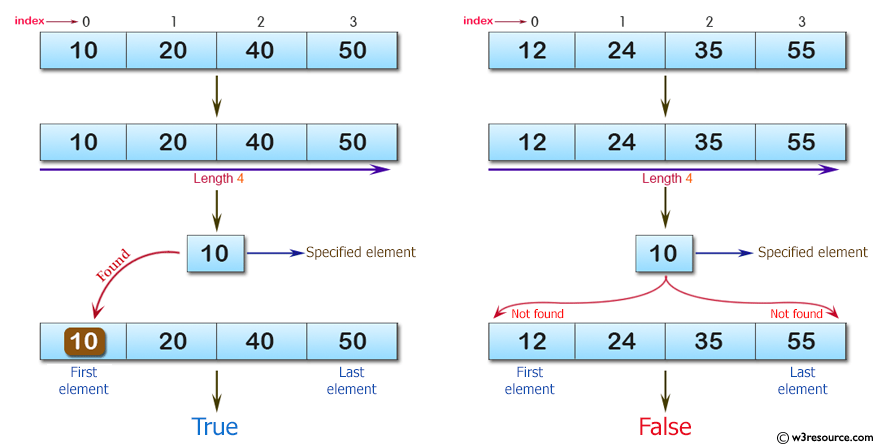
Sample Solution:-
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
// Entry point of the program
static void Main(string[] args)
{
// Calling the test method with different integer arrays and printing the results
Console.WriteLine(test(new[] { 10, 20, 40, 50 }));
Console.WriteLine(test(new[] { 5, 20, 40, 10 }));
Console.WriteLine(test(new[] { 10, 20, 40, 10 }));
Console.WriteLine(test(new[] { 12, 24, 35, 55 }));
// Keeps the console window open until a key is pressed
Console.ReadLine();
}
// Method to check if the first or last element of the array is equal to 10
public static bool test(int[] nums)
{
// Returns true if the first element is equal to 10 or the last element is equal to 10
return nums[0] == 10 || nums[nums.Length - 1] == 10;
}
}
}
Sample Output:
True True True False
Flowchart:
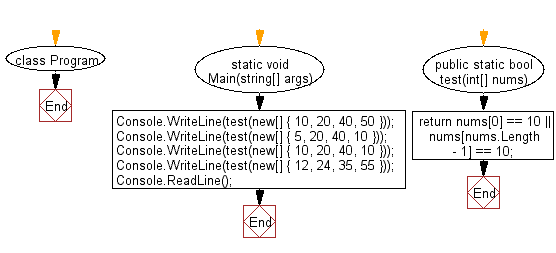
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string from a given string. If the first or first two characters is 'a', return the string without those 'a' characters otherwise return the original given string.
Next: Write a C# Sharp program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-88.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics