C#: Find out the maximum element between the first or last element in a given array of integers
C# Sharp Basic Algorithm: Exercise-94 with Solution
Replace All Elements with Max of First/Last
Write a C# Sharp program to find out the maximum element between the first and last element in a given array of integers ( length 4), replace all elements with the maximum element.
Visual Presentation:
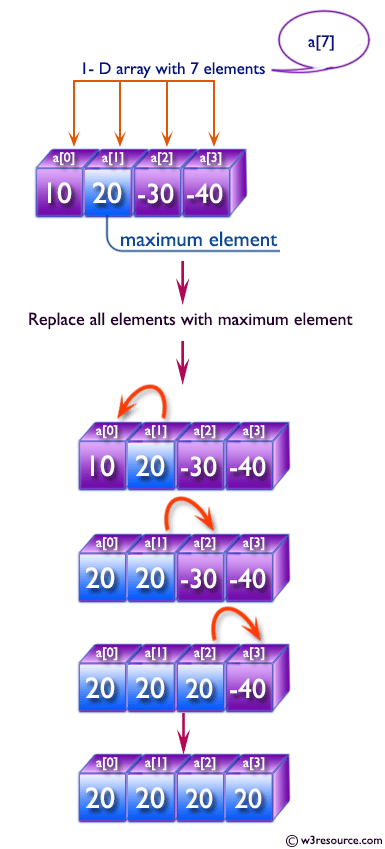
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
using System.Linq; // Importing the System.Linq namespace for LINQ functionalities
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, -30, -40 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach (var i in item)
{
Console.Write(i.ToString() + " "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to create a new array filled with the maximum value of the given array's elements
public static int[] test(int[] nums)
{
int max = nums.Max(); // Finding the maximum value in the 'nums' array using LINQ's Max() method
// Returning a new array with all elements set to the maximum value found above
return new int[] { max, max, max, max };
}
}
}
Sample Output:
New array: 20 20 20 20
Flowchart:
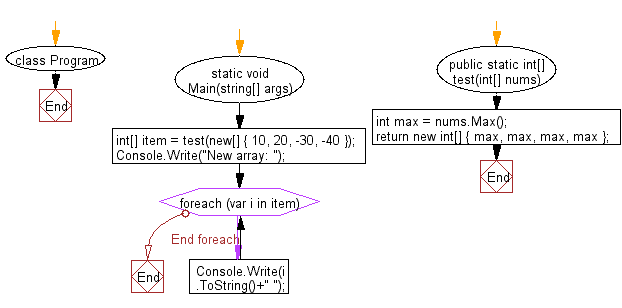
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to reverse a given array of integers and length 4.
Next: Write a C# Sharp program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-94.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics