C#: Print hello and your name in a separate line
Print Hello and Name
Write a C# Sharp program to print Hello and your name in a separate line.
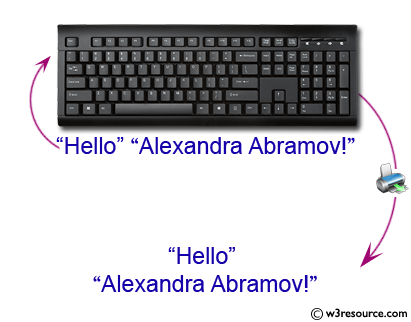
Sample Solution:
C# Sharp Code:
// This is the beginning of the Exercise1 class
public class Exercise1
{
// This is the main method where the program execution starts
public static void Main()
{
// This line outputs "Hello" to the console
System.Console.WriteLine("Hello");
// This line outputs "Alexandra Abramov!" to the console
System.Console.WriteLine("Alexandra Abramov!");
}
}
Sample Output:
Hello Alexandra Abramov!
System.Console.WriteLine method writes the specified data, followed by the current line terminator, to the standard output stream.
Details of WriteLine() Method:
Methods | Description |
---|---|
WriteLine() | This method writes the current line terminator to the standard output stream. |
WriteLine(Boolean) | This method writes the text representation of the specified Boolean value, followed by the current line terminator, to the standard output stream. |
WriteLine(Char) | This method writes the specified Unicode character, followed by the current line terminator, value to the standard output stream. |
WriteLine(Char[]) | This method writes the specified array of Unicode characters, followed by the current line terminator, to the standard output stream. |
WriteLine(Char[], Int32, Int32) | This method writes the specified subarray of Unicode characters, followed by the current line terminator, to the standard output stream. |
WriteLine(Decimal) | This method writes the text representation of the specified Decimal value, followed by the current line terminator, to the standard output stream. |
WriteLine(Double) | This method writes the text representation of the specified double-precision floating-point value, followed by the current line terminator, to the standard output stream. |
WriteLine(Int32) | This method writes the text representation of the specified 32-bit signed integer value, followed by the current line terminator, to the standard output stream. |
WriteLine(Int64) | This method writes the text representation of the specified 64-bit signed integer value, followed by the current line terminator, to the standard output stream. |
WriteLine(Object) | This method writes the text representation of the specified object, followed by the current line terminator, to the standard output stream. |
WriteLine(Single) | This method writes the text representation of the specified single-precision floating-point value, followed by the current line terminator, to the standard output stream. |
WriteLine(String) | This method writes the specified string value, followed by the current line terminator, to the standard output stream. |
WriteLine(String,Object) | This method writes the text representation of the specified object, followed by the current line terminator, to the standard output stream using the specified format information. |
WriteLine(String,Object,Object) | This method writes the text representation of the specified objects, followed by the current line terminator, to the standard output stream using the specified format information. |
WriteLine(String,Object,Object,Object) | This method writes the text representation of the specified objects, followed by the current line terminator, to the standard output stream using the specified format information. |
WriteLine(String,Object,Object,Object,Object) | This method writes the text representation of the specified objects and variable-length parameter list, followed by the current line terminator, to the standard output stream using the specified format information. |
WriteLine(String,Object[]) | This method writes the text representation of the specified array of objects, followed by the current line terminator, to the standard output stream using the specified format information. |
WriteLine(UInt32) | This method writes the text representation of the specified 32-bit unsigned integer value, followed by the current line terminator, to the standard output stream. |
WriteLine(UInt64) | This method writes the text representation of the specified 64-bit unsigned integer value, followed by the current line terminator, to the standard output stream. |
Flowchart:
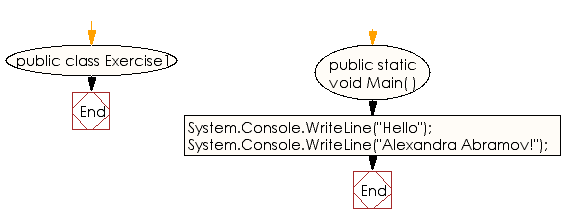
C# Sharp Code Editor:
Previous: C# Sharp Basic Exercises.
Next: Write a C# Sharp program to print the sum of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.