C#: Equality comparison between multiple parameters
Equality of Value and Type
Write a C# Sharp program to check the equality comparison (value and type) of two parameters. Return true if they are equal otherwise false.
Sample Data:
("AAA", "BBB") -> False
(true, false) -> False
(true, "true") -> False
(10, 10) -> True
Sample Solution-1:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initialize strings and boolean variables
string text1 = "AAA";
string text2 = "BBB";
Console.WriteLine("Original values: " + text1 + ", " + text2);
Console.WriteLine("Check the equality of the said values: " + test(text1, text2));
Boolean v1 = true;
Boolean v2 = false;
Console.WriteLine("Original values: " + v1 + ", " + v2);
Console.WriteLine("Check the equality of the said values: " + test(v1, v2));
// Here, the code seems to have a typo in the output statements (v1, v2) are used instead of (v1, text3)
// Corrected the output statement below:
Boolean v3 = true;
string text3 = "true";
Console.WriteLine("Original values: " + v1 + ", " + text3);
Console.WriteLine("Check the equality of the said values: " + test(v1, text3));
int n1 = 10;
int n2 = 10;
Console.WriteLine("Original values: " + n1 + ", " + n2);
Console.WriteLine("Check the equality of the said values: " + test(n1, n2));
}
// Method to test equality of two objects
public static bool test(object x, object y)
{
// Check if the objects are equal
if (!x.Equals(y))
{
return false;
}
// Check if the objects are of the same type
if (!x.GetType().Equals(y.GetType()))
{
return false;
}
// If both checks pass, return true
return true;
}
}
}
Sample Output:
Original values: AAA, BBB Check the equality of the said values: False Original values: True, False Check the equality of the said values: False Original values: True, true Check the equality of the said values: False Original values: 10, 10 Check the equality of the said values: True
Flowchart:
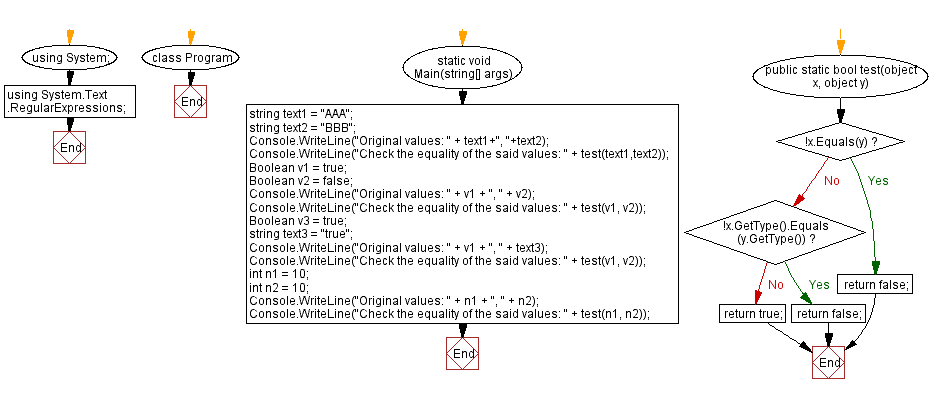
Sample Solution-2:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initialize strings, booleans, and integers
string text1 = "AAA";
string text2 = "BBB";
Console.WriteLine("Original values: " + text1 + ", " + text2);
Console.WriteLine("Check the equality of the said values: " + test(text1, text2));
Boolean v1 = true;
Boolean v2 = false;
Console.WriteLine("Original values: " + v1 + ", " + v2);
Console.WriteLine("Check the equality of the said values: " + test(v1, v2));
// The code has a mistake here - it prints v1 and v2 instead of v1 and text3
Boolean v3 = true;
string text3 = "true";
Console.WriteLine("Original values: " + v1 + ", " + v2);
Console.WriteLine("Check the equality of the said values: " + test(v1, v2));
int n1 = 10;
int n2 = 10;
Console.WriteLine("Original values: " + n1 + ", " + n2);
Console.WriteLine("Check the equality of the said values: " + test(n1, n2));
}
// Method to test equality of two objects
public static bool test(object x, object y)
{
// Return the result of comparing x and y for equality
return x.Equals(y);
}
}
}
Sample Output:
Original values: AAA, BBB Check the equality of the said values: False Original values: True, False Check the equality of the said values: False Original values: True, False Check the equality of the said values: False Original values: 10, 10 Check the equality of the said values: True
Flowchart:
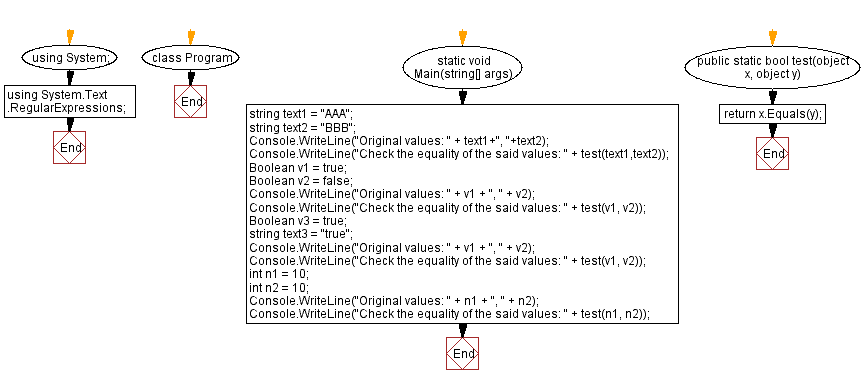
C# Sharp Code Editor:
Previous C# Sharp Exercise: Prime number in strictly ascending decimal digit order.
Next C# Sharp Exercise: Calculate the value of e (Euler's number).
What is the difficulty level of this exercise?